NPS analysis
NPS - Comment analysis
In an [previous post](https://nitinahuja.github.io/2017/nps-exploratory-analysis-in-r/) we performed some EDA on the NPS data we have. Recall that as part of the question about the likelihood of recommending a service or business there is an optional text response about why they picked this score.
Let’s try and see what those responses are all about. We had already performed some sentiment analysis on this text we are now going to attempt to classify this text into topics. Topic modeling is a method for unsupervised classification of such documents, similar to clustering on numeric data, which finds natural groups of items even when we’re not sure what we’re looking for.
Latent Dirichlet allocation (LDA) is a particularly popular method for fitting a topic model. It treats each document as a mixture of topics, and each topic as a mixture of words.
LDA has two key tenets
- Every document is a mixture of topics
- Every topic is a mixture of words.
In our NPS comment data, we will treat each comment as a document and we’ll try and see how many topics we can identify. Since LDA is unsupervised classification, and we do not have any a priori knowledge of the possible number of topics, we’ll need to do some trial and error.
Let’s start by loading the required libraries
library(irlba)
library(tidytext)
library(topicmodels)
library(ggplot2)
library(tidyr)
library(dplyr)
library(RColorBrewer)
library(lubridate)
library(stringr)
library(widyr)
library(broom)
library(tidygraph)
library(ggraph)
library(ggrepel)
LDA needs a term document matrix, let’s start by reading in the data and remove the rows that do not have any comments.
nps <- read.csv('~/data/nps.csv', header = TRUE, quote = '"'
, na.strings=c("", "NA", "#N/A")
, colClasses = c("Feedback.Received"= "Date"
, "Comment"="character"
, "Survey.Sent"="Date"
, "TPY"="integer"
))
nps<- nps %>% drop_na(Comment)
That leaves us with 1130 rows.
Let’s also enrich the data with some additional fields and add the category like we did in the last post. The category is dependent on the score and scores from 0 through 6 are considered detractors, 7 - 8 are passives and 9 and 10 are promorters.
nps$year <- as.factor(year(nps$Received))
nps$month <- cut(nps$Received, breaks = "month")
nps$days <- nps$Received - nps$Sent
nps$weekday <- weekdays(nps$Received)
nps$weekday <- factor(nps$weekday, levels = c("Sunday", "Monday","Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"))
nps$cat <- cut(nps$Score, breaks = c(-1,6,8,10), labels = c("Detractor", "Passive", "Promoter"))
Extract out the comments while keeping the category
comments <- nps %>%
select(Comment, cat) %>%
group_by(row_number())
LDA using per comment as a document
The LDA function takes a TermDocumentMatrix object as an input; let’s convert our text into a DFM
nps_count <- comments %>%
unnest_tokens(word, Comment) %>%
anti_join(stop_words, by = c('word')) %>%
count(`row_number()`, word, sort = TRUE) %>%
ungroup()
Now convert to a DTM and perform the LDA
nps_dtm <- nps_count %>%
cast_dtm(`row_number()`, word, n)
LDA on the matrix. This produces a row per topic with each word and its probability of the word being generated by that topic. We picked seven topics but we will experiment with a few more combinations.
topic_count <- 25
nps_lda <- LDA(nps_dtm, k = topic_count, control = list(seed=1234))
nps_topics <- tidy(nps_lda, matrix = "beta")
Next - let’s find out the top 5 words per topic and plot those.
top_terms <- nps_topics %>%
group_by(topic) %>%
top_n(5, beta) %>%
ungroup() %>%
arrange(topic, -beta)
Plot the terms and topics.
top_terms %>%
mutate(term = reorder(term, beta)) %>%
ggplot(aes(term, beta, fill=factor(topic))) +
geom_col(show.legend = FALSE) +
facet_wrap(~ topic, scales = "free" ) +
coord_flip() +
guides(fill=FALSE) +
labs(title = "Terms in topics - By Comment", x = "Term", y = "Probability")
## Warning: The `<scale>` argument of `guides()` cannot be `FALSE`. Use "none" instead as
## of ggplot2 3.3.4.
## This warning is displayed once every 8 hours.
## Call `lifecycle::last_lifecycle_warnings()` to see where this warning was
## generated.
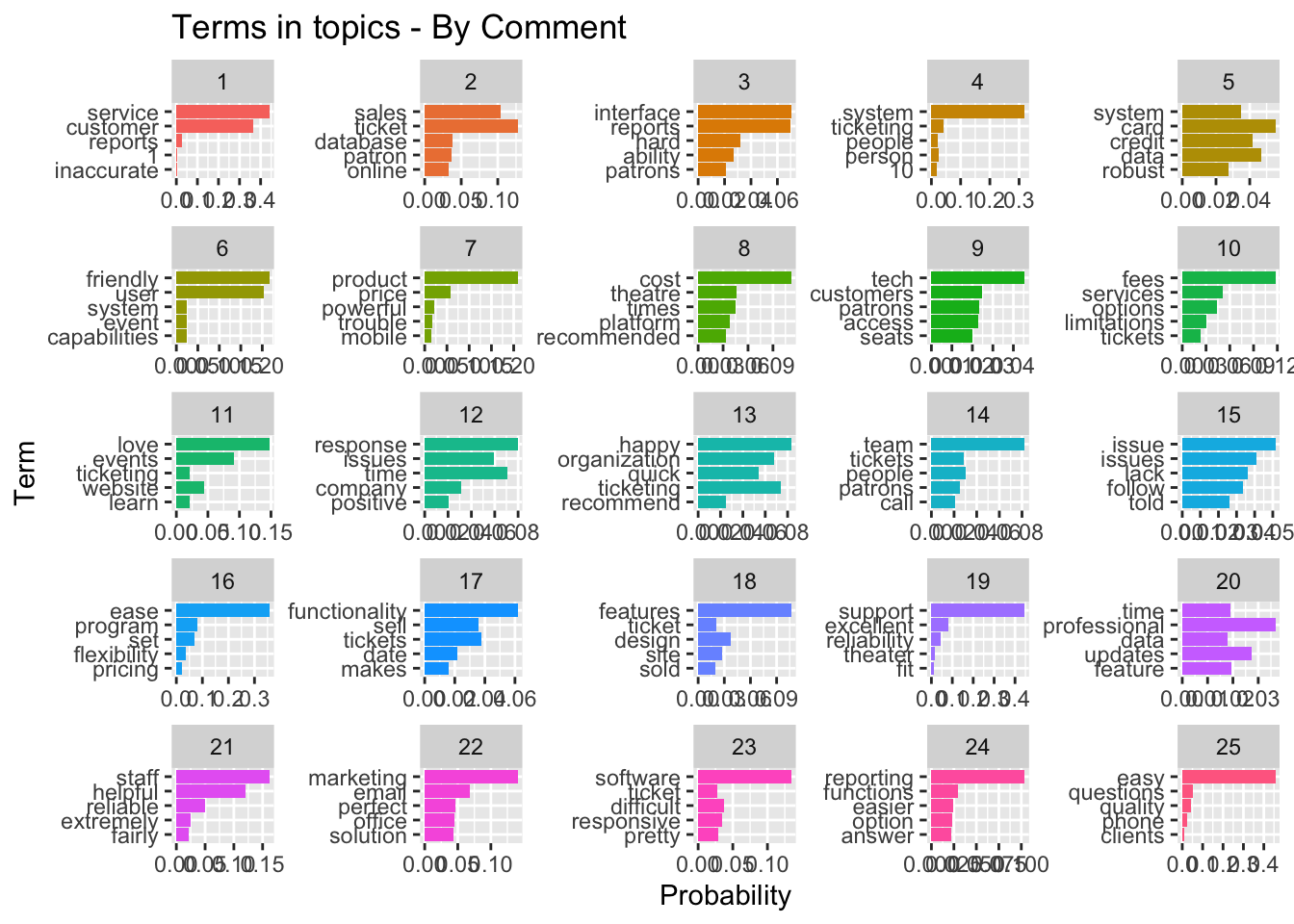
In this model, we treated each comment as it’s own document and picked 20 topics to classify the text, we assume that each responder roughly commented across the same 20 topics; how does this change if we treat each category as it’s own document. Are respondents per category talking about the same topics.
LDA By Category
There are three categories and we will try to classify the text into three topics but this time let’s try to use bigrams for this.
nps_count <- comments %>%
ungroup() %>%
unnest_tokens(word ,Comment, token = "ngrams", n = 2) %>%
count(cat, word, sort = TRUE) %>%
ungroup()
Now convert to a DTM and perform the LDA
nps_dtm <- nps_count %>%
cast_dtm(cat, word, n)
*LDA on the matrix. *
This produces a row per topic with each word and its probability of the word being generated by that topic. We picked three topics but we will experiment with a few more combinations.
nps_lda <- LDA(nps_dtm, k = 3, control = list(seed=1234))
nps_topics <- tidy(nps_lda, matrix = "beta")
Next - let’s find out the top words per topic and plot those.
top_terms <- nps_topics %>%
group_by(topic) %>%
top_n(10, beta) %>%
ungroup() %>%
arrange(topic, -beta)
Plot the bigrams and topics; looking at the top terms, these seem to map well to the three categories - Promoters, Passives and detractors as 1, 2 and 3 respectively.
top_terms %>%
mutate(term = reorder(term, beta)) %>%
ggplot(aes(term, beta, fill=factor(topic))) +
geom_col(show.legend = FALSE) +
facet_wrap( ~ topic, scales = "free" ) +
coord_flip() +
guides(fill=FALSE) +
labs(title = "Bigrams in topics - By Category", x = "Term", y = "Probability")
## Warning: The `<scale>` argument of `guides()` cannot be `FALSE`. Use "none" instead as
## of ggplot2 3.3.4.
## This warning is displayed once every 8 hours.
## Call `lifecycle::last_lifecycle_warnings()` to see where this warning was
## generated.
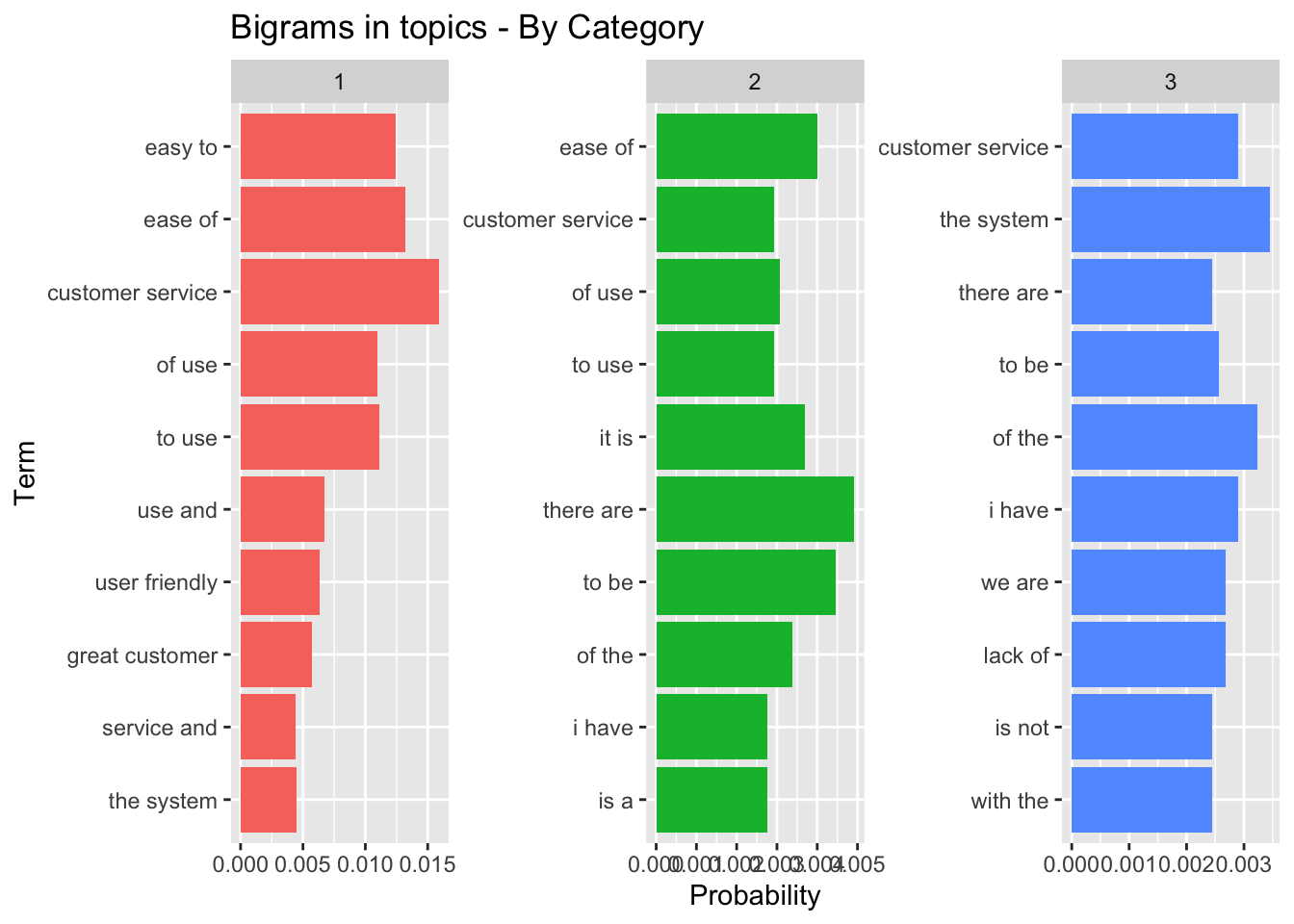
LDA can also model each document as a mixture of topics. We can examine the per-document-per-topic probabilities, called Y (“gamma”), with the matrix = “gamma” argument to tidy()
nps_docs <- tidy(nps_lda, matrix = "gamma")
nps_docs
## # A tibble: 9 × 3
## document topic gamma
## <chr> <int> <dbl>
## 1 Detractor 1 0.00000351
## 2 Passive 1 0.00000485
## 3 Promoter 1 1.00
## 4 Detractor 2 0.00000351
## 5 Passive 2 1.00
## 6 Promoter 2 0.00000417
## 7 Detractor 3 1.00
## 8 Passive 3 0.00000485
## 9 Promoter 3 0.00000417
Each of these values is an estimated proportion of words from that document that are generated from that topic. This confirms what we suspected - that topic 1 words were almost entirely (99%) generated of words from the promoters sections and topic 2’s words were generated from the passive documents.
Zipf’s Law
Let’s take a short detour before moving ahead. George Zipf, in the 1940’s, by hand took all the words in Ulysses and found that the frequency of a word was inversely proportinal to it’s rank. This observation applies to many phenomenon, including populations in cities.
nps_count <- nps %>%
select(Comment, cat) %>%
unnest_tokens(word, Comment) %>%
count(cat, word, sort = TRUE) %>%
ungroup()
cat_count <- nps_count %>%
group_by(cat) %>%
summarise( total = sum(n))
nps_count <- left_join(nps_count, cat_count, by = 'cat')
freq_by_rank <- nps_count %>%
group_by(cat) %>%
mutate(rank = row_number(), freq = n/total)
Now let’s plot the log-log plot for the rank and frequency.
freq_by_rank %>%
ggplot(aes(rank, freq, color = cat)) +
geom_line() +
scale_x_log10() +
scale_y_log10() +
scale_fill_brewer("category", palette = "Set1") +
labs(title = "Zipf's law")
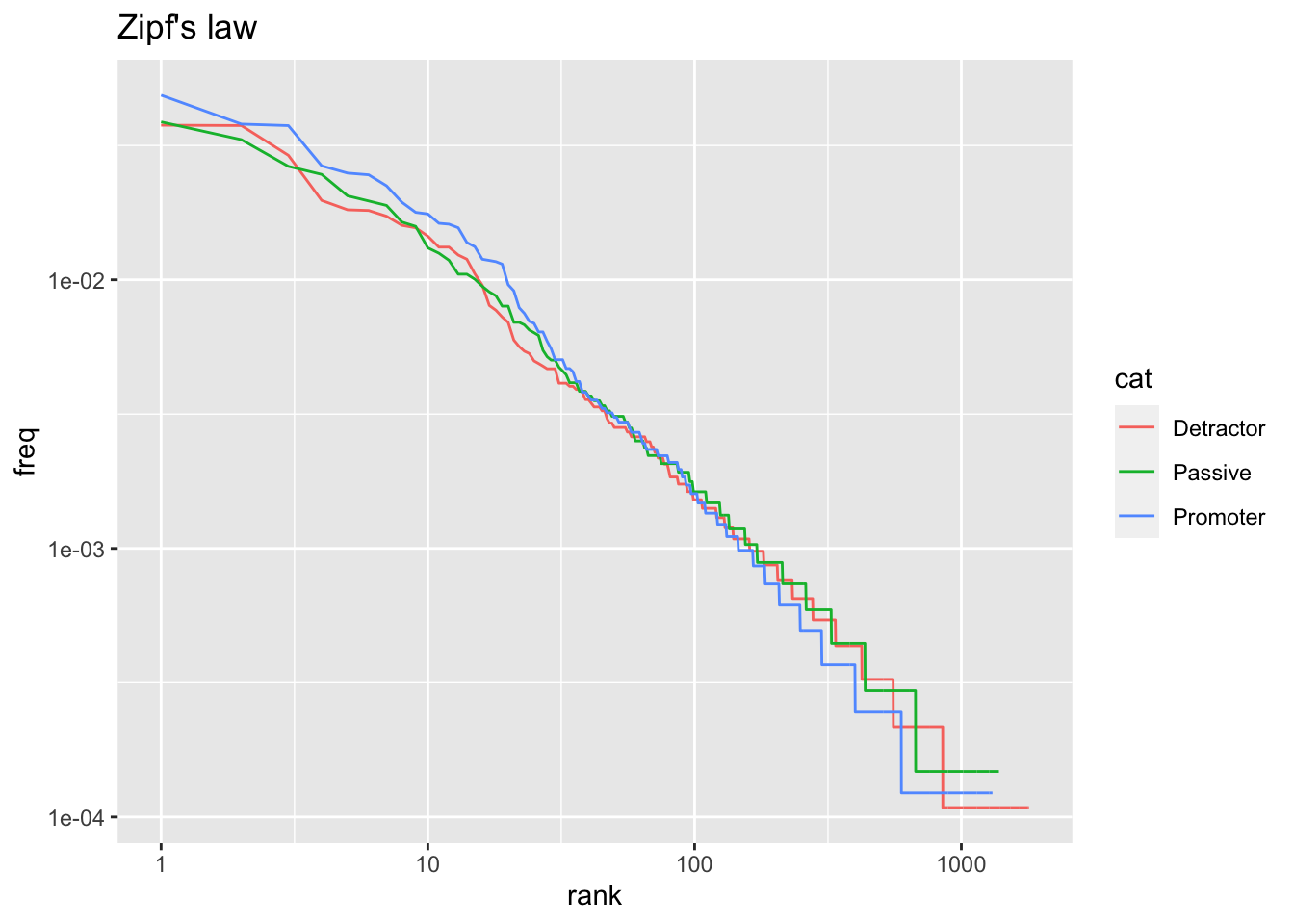
It’s quite amazing to see that even a fragmented set of comments follows Zipf’s law. The slope is not quite -1 but close. We can find out what the linear fit is. Really close to -1.
lm(log10(freq) ~ log10(rank), data = freq_by_rank %>% filter(rank > 10))
##
## Call:
## lm(formula = log10(freq) ~ log10(rank), data = freq_by_rank %>%
## filter(rank > 10))
##
## Coefficients:
## (Intercept) log10(rank)
## -0.7715 -1.0264
Word Vectors
Word vectors are typically calculated using neural networks; that is what word2vec is. We will try to find word vectors in our own collection using some linear algebra. A lot of this is influenced by the excellent post by Julia Silge
We’ll start by counting unigram probabilities
comments <- nps %>%
select(Comment, cat) %>%
mutate(docID = row_number())
unigram_probs <- comments %>%
unnest_tokens(word, Comment) %>%
count(word, sort = TRUE) %>%
mutate(p = n /sum(n))
unigram_probs
## word n p
## 1 the 913 3.785084e-02
## 2 to 881 3.652419e-02
## 3 and 843 3.494880e-02
## 4 of 523 2.168235e-02
## 5 is 503 2.085320e-02
## 6 a 481 1.994113e-02
## 7 i 418 1.732930e-02
## 8 for 367 1.521496e-02
## 9 it 312 1.293479e-02
## 10 we 301 1.247875e-02
## 11 have 293 1.214709e-02
## 12 service 280 1.160814e-02
## 13 use 274 1.135940e-02
## 14 that 268 1.111065e-02
## 15 with 264 1.094482e-02
## 16 are 242 1.003275e-02
## 17 customer 229 9.493802e-03
## 18 our 225 9.327971e-03
## 19 in 211 8.747564e-03
## 20 not 209 8.664649e-03
## 21 great 201 8.332988e-03
## 22 system 191 7.918411e-03
## 23 support 187 7.752581e-03
## 24 easy 155 6.425936e-03
## 25 be 154 6.384478e-03
## 26 but 143 5.928444e-03
## 27 ease 142 5.886986e-03
## 28 on 137 5.679698e-03
## 29 very 134 5.555325e-03
## 30 there 125 5.182206e-03
## 31 you 120 4.974918e-03
## 32 would 118 4.892003e-03
## 33 been 114 4.726172e-03
## 34 as 112 4.643257e-03
## 35 like 112 4.643257e-03
## 36 has 104 4.311596e-03
## 37 your 96 3.979934e-03
## 38 when 93 3.855562e-03
## 39 ticket 91 3.772646e-03
## 40 friendly 89 3.689731e-03
## 41 good 89 3.689731e-03
## 42 user 84 3.482443e-03
## 43 my 82 3.399527e-03
## 44 an 79 3.275154e-03
## 45 all 77 3.192239e-03
## 46 tickets 77 3.192239e-03
## 47 do 73 3.026409e-03
## 48 from 73 3.026409e-03
## 49 or 71 2.943493e-03
## 50 this 71 2.943493e-03
## 51 up 70 2.902036e-03
## 52 it's 69 2.860578e-03
## 53 some 68 2.819120e-03
## 54 ticketing 68 2.819120e-03
## 55 they 66 2.736205e-03
## 56 needs 65 2.694747e-03
## 57 product 65 2.694747e-03
## 58 us 65 2.694747e-03
## 59 more 64 2.653290e-03
## 60 so 63 2.611832e-03
## 61 get 62 2.570374e-03
## 62 if 61 2.528917e-03
## 63 no 61 2.528917e-03
## 64 patrons 61 2.528917e-03
## 65 need 59 2.446001e-03
## 66 email 58 2.404544e-03
## 67 other 57 2.363086e-03
## 68 at 56 2.321628e-03
## 69 can 55 2.280171e-03
## 70 reports 55 2.280171e-03
## 71 work 55 2.280171e-03
## 72 one 54 2.238713e-03
## 73 issues 53 2.197256e-03
## 74 works 53 2.197256e-03
## 75 sales 52 2.155798e-03
## 76 was 52 2.155798e-03
## 77 always 51 2.114340e-03
## 78 had 51 2.114340e-03
## 79 love 51 2.114340e-03
## 80 marketing 51 2.114340e-03
## 81 don't 50 2.072883e-03
## 82 software 50 2.072883e-03
## 83 staff 50 2.072883e-03
## 84 way 50 2.072883e-03
## 85 also 48 1.989967e-03
## 86 time 48 1.989967e-03
## 87 many 47 1.948510e-03
## 88 patron 46 1.907052e-03
## 89 because 44 1.824137e-03
## 90 events 44 1.824137e-03
## 91 too 44 1.824137e-03
## 92 help 43 1.782679e-03
## 93 well 43 1.782679e-03
## 94 fees 42 1.741221e-03
## 95 able 41 1.699764e-03
## 96 features 40 1.658306e-03
## 97 helpful 40 1.658306e-03
## 98 program 40 1.658306e-03
## 99 things 39 1.616848e-03
## 100 about 38 1.575391e-03
## 101 high 38 1.575391e-03
## 102 me 38 1.575391e-03
## 103 problems 38 1.575391e-03
## 104 excellent 37 1.533933e-03
## 105 only 37 1.533933e-03
## 106 still 37 1.533933e-03
## 107 10 36 1.492475e-03
## 108 reporting 36 1.492475e-03
## 109 their 36 1.492475e-03
## 110 were 36 1.492475e-03
## 111 most 35 1.451018e-03
## 112 what 35 1.451018e-03
## 113 better 34 1.409560e-03
## 114 data 33 1.368102e-03
## 115 set 33 1.368102e-03
## 116 any 32 1.326645e-03
## 117 new 32 1.326645e-03
## 118 people 32 1.326645e-03
## 119 years 32 1.326645e-03
## 120 cost 31 1.285187e-03
## 121 could 31 1.285187e-03
## 122 how 31 1.285187e-03
## 123 just 31 1.285187e-03
## 124 team 31 1.285187e-03
## 125 than 31 1.285187e-03
## 126 using 31 1.285187e-03
## 127 which 31 1.285187e-03
## 128 everything 30 1.243730e-03
## 129 functionality 30 1.243730e-03
## 130 really 30 1.243730e-03
## 131 can't 29 1.202272e-03
## 132 event 29 1.202272e-03
## 133 few 29 1.202272e-03
## 134 make 29 1.202272e-03
## 135 online 29 1.202272e-03
## 136 organization 29 1.202272e-03
## 137 am 28 1.160814e-03
## 138 see 28 1.160814e-03
## 139 happy 27 1.119357e-03
## 140 year 27 1.119357e-03
## 141 2 26 1.077899e-03
## 142 best 26 1.077899e-03
## 143 by 26 1.077899e-03
## 144 much 26 1.077899e-03
## 145 never 26 1.077899e-03
## 146 now 26 1.077899e-03
## 147 out 26 1.077899e-03
## 148 response 26 1.077899e-03
## 149 theatre 26 1.077899e-03
## 150 being 25 1.036441e-03
## 151 call 25 1.036441e-03
## 152 interface 25 1.036441e-03
## 153 lack 25 1.036441e-03
## 154 company 24 9.949836e-04
## 155 difficult 24 9.949836e-04
## 156 issue 24 9.949836e-04
## 157 who 24 9.949836e-04
## 158 will 24 9.949836e-04
## 159 into 23 9.535260e-04
## 160 office 23 9.535260e-04
## 161 over 23 9.535260e-04
## 162 them 23 9.535260e-04
## 163 different 22 9.120683e-04
## 164 find 22 9.120683e-04
## 165 however 22 9.120683e-04
## 166 overall 22 9.120683e-04
## 167 perfect 22 9.120683e-04
## 168 card 21 8.706107e-04
## 169 even 21 8.706107e-04
## 170 give 21 8.706107e-04
## 171 having 21 8.706107e-04
## 172 made 21 8.706107e-04
## 173 management 21 8.706107e-04
## 174 needed 21 8.706107e-04
## 175 options 21 8.706107e-04
## 176 price 21 8.706107e-04
## 177 problem 21 8.706107e-04
## 178 questions 21 8.706107e-04
## 179 seems 21 8.706107e-04
## 180 services 21 8.706107e-04
## 181 several 21 8.706107e-04
## 182 database 20 8.291530e-04
## 183 does 20 8.291530e-04
## 184 donation 20 8.291530e-04
## 185 each 20 8.291530e-04
## 186 know 20 8.291530e-04
## 187 number 20 8.291530e-04
## 188 small 20 8.291530e-04
## 189 times 20 8.291530e-04
## 190 website 20 8.291530e-04
## 191 back 19 7.876954e-04
## 192 box 19 7.876954e-04
## 193 credit 19 7.876954e-04
## 194 feature 19 7.876954e-04
## 195 little 19 7.876954e-04
## 196 reliability 19 7.876954e-04
## 197 report 19 7.876954e-04
## 198 should 19 7.876954e-04
## 199 someone 19 7.876954e-04
## 200 want 19 7.876954e-04
## 201 working 19 7.876954e-04
## 202 1 18 7.462377e-04
## 203 ability 18 7.462377e-04
## 204 business 18 7.462377e-04
## 205 change 18 7.462377e-04
## 206 donations 18 7.462377e-04
## 207 e 18 7.462377e-04
## 208 go 18 7.462377e-04
## 209 higher 18 7.462377e-04
## 210 intuitive 18 7.462377e-04
## 211 lot 18 7.462377e-04
## 212 order 18 7.462377e-04
## 213 reliable 18 7.462377e-04
## 214 sell 18 7.462377e-04
## 215 take 18 7.462377e-04
## 216 after 17 7.047801e-04
## 217 changes 17 7.047801e-04
## 218 customers 17 7.047801e-04
## 219 doesn't 17 7.047801e-04
## 220 flexibility 17 7.047801e-04
## 221 i've 17 7.047801e-04
## 222 last 17 7.047801e-04
## 223 process 17 7.047801e-04
## 224 quick 17 7.047801e-04
## 225 recommend 17 7.047801e-04
## 226 score 17 7.047801e-04
## 227 think 17 7.047801e-04
## 228 through 17 7.047801e-04
## 229 without 17 7.047801e-04
## 230 available 16 6.633224e-04
## 231 extremely 16 6.633224e-04
## 232 getting 16 6.633224e-04
## 233 i'm 16 6.633224e-04
## 234 makes 16 6.633224e-04
## 235 phone 16 6.633224e-04
## 236 robust 16 6.633224e-04
## 237 solution 16 6.633224e-04
## 238 tech 16 6.633224e-04
## 239 add 15 6.218648e-04
## 240 answer 15 6.218648e-04
## 241 bit 15 6.218648e-04
## 242 emails 15 6.218648e-04
## 243 every 15 6.218648e-04
## 244 expensive 15 6.218648e-04
## 245 long 15 6.218648e-04
## 246 nice 15 6.218648e-04
## 247 often 15 6.218648e-04
## 248 person 15 6.218648e-04
## 249 quality 15 6.218648e-04
## 250 same 15 6.218648e-04
## 251 show 15 6.218648e-04
## 252 since 15 6.218648e-04
## 253 those 15 6.218648e-04
## 254 told 15 6.218648e-04
## 255 two 15 6.218648e-04
## 256 while 15 6.218648e-04
## 257 design 14 5.804071e-04
## 258 donor 14 5.804071e-04
## 259 first 14 5.804071e-04
## 260 glitches 14 5.804071e-04
## 261 improvements 14 5.804071e-04
## 262 multiple 14 5.804071e-04
## 263 platform 14 5.804071e-04
## 264 sometimes 14 5.804071e-04
## 265 we've 14 5.804071e-04
## 266 where 14 5.804071e-04
## 267 yet 14 5.804071e-04
## 268 both 13 5.389495e-04
## 269 contact 13 5.389495e-04
## 270 day 13 5.389495e-04
## 271 easier 13 5.389495e-04
## 272 especially 13 5.389495e-04
## 273 etc 13 5.389495e-04
## 274 functions 13 5.389495e-04
## 275 information 13 5.389495e-04
## 276 keep 13 5.389495e-04
## 277 limitations 13 5.389495e-04
## 278 members 13 5.389495e-04
## 279 offer 13 5.389495e-04
## 280 offers 13 5.389495e-04
## 281 others 13 5.389495e-04
## 282 pretty 13 5.389495e-04
## 283 purchase 13 5.389495e-04
## 284 put 13 5.389495e-04
## 285 reason 13 5.389495e-04
## 286 recommended 13 5.389495e-04
## 287 responsive 13 5.389495e-04
## 288 s 13 5.389495e-04
## 289 seat 13 5.389495e-04
## 290 simple 13 5.389495e-04
## 291 site 13 5.389495e-04
## 292 though 13 5.389495e-04
## 293 used 13 5.389495e-04
## 294 wish 13 5.389495e-04
## 295 agent 12 4.974918e-04
## 296 based 12 4.974918e-04
## 297 before 12 4.974918e-04
## 298 certain 12 4.974918e-04
## 299 companies 12 4.974918e-04
## 300 did 12 4.974918e-04
## 301 experience 12 4.974918e-04
## 302 flexible 12 4.974918e-04
## 303 hard 12 4.974918e-04
## 304 look 12 4.974918e-04
## 305 looking 12 4.974918e-04
## 306 may 12 4.974918e-04
## 307 per 12 4.974918e-04
## 308 professional 12 4.974918e-04
## 309 received 12 4.974918e-04
## 310 season 12 4.974918e-04
## 311 selling 12 4.974918e-04
## 312 technical 12 4.974918e-04
## 313 theater 12 4.974918e-04
## 314 access 11 4.560342e-04
## 315 account 11 4.560342e-04
## 316 along 11 4.560342e-04
## 317 cannot 11 4.560342e-04
## 318 clients 11 4.560342e-04
## 319 done 11 4.560342e-04
## 320 end 11 4.560342e-04
## 321 fantastic 11 4.560342e-04
## 322 fee 11 4.560342e-04
## 323 feel 11 4.560342e-04
## 324 follow 11 4.560342e-04
## 325 inability 11 4.560342e-04
## 326 ipad 11 4.560342e-04
## 327 less 11 4.560342e-04
## 328 mail 11 4.560342e-04
## 329 navigate 11 4.560342e-04
## 330 non 11 4.560342e-04
## 331 nothing 11 4.560342e-04
## 332 orders 11 4.560342e-04
## 333 single 11 4.560342e-04
## 334 track 11 4.560342e-04
## 335 tracking 11 4.560342e-04
## 336 worked 11 4.560342e-04
## 337 3 10 4.145765e-04
## 338 capabilities 10 4.145765e-04
## 339 care 10 4.145765e-04
## 340 computer 10 4.145765e-04
## 341 ever 10 4.145765e-04
## 342 fact 10 4.145765e-04
## 343 fixed 10 4.145765e-04
## 344 found 10 4.145765e-04
## 345 given 10 4.145765e-04
## 346 handle 10 4.145765e-04
## 347 packages 10 4.145765e-04
## 348 receive 10 4.145765e-04
## 349 satisfied 10 4.145765e-04
## 350 say 10 4.145765e-04
## 351 seats 10 4.145765e-04
## 352 seem 10 4.145765e-04
## 353 such 10 4.145765e-04
## 354 thank 10 4.145765e-04
## 355 tool 10 4.145765e-04
## 356 tools 10 4.145765e-04
## 357 updates 10 4.145765e-04
## 358 ways 10 4.145765e-04
## 359 wonderful 10 4.145765e-04
## 360 4 9 3.731189e-04
## 361 again 9 3.731189e-04
## 362 amazing 9 3.731189e-04
## 363 anything 9 3.731189e-04
## 364 around 9 3.731189e-04
## 365 client 9 3.731189e-04
## 366 create 9 3.731189e-04
## 367 development 9 3.731189e-04
## 368 fairly 9 3.731189e-04
## 369 fix 9 3.731189e-04
## 370 function 9 3.731189e-04
## 371 helps 9 3.731189e-04
## 372 i'd 9 3.731189e-04
## 373 important 9 3.731189e-04
## 374 instead 9 3.731189e-04
## 375 its 9 3.731189e-04
## 376 mobile 9 3.731189e-04
## 377 money 9 3.731189e-04
## 378 name 9 3.731189e-04
## 379 off 9 3.731189e-04
## 380 organizations 9 3.731189e-04
## 381 pricing 9 3.731189e-04
## 382 print 9 3.731189e-04
## 383 processing 9 3.731189e-04
## 384 purchases 9 3.731189e-04
## 385 requests 9 3.731189e-04
## 386 run 9 3.731189e-04
## 387 said 9 3.731189e-04
## 388 sale 9 3.731189e-04
## 389 setting 9 3.731189e-04
## 390 shows 9 3.731189e-04
## 391 slow 9 3.731189e-04
## 392 sold 9 3.731189e-04
## 393 something 9 3.731189e-04
## 394 useful 9 3.731189e-04
## 395 venues 9 3.731189e-04
## 396 25 8 3.316612e-04
## 397 addresses 8 3.316612e-04
## 398 ago 8 3.316612e-04
## 399 already 8 3.316612e-04
## 400 another 8 3.316612e-04
## 401 app 8 3.316612e-04
## 402 areas 8 3.316612e-04
## 403 basic 8 3.316612e-04
## 404 constantly 8 3.316612e-04
## 405 convenience 8 3.316612e-04
## 406 crm 8 3.316612e-04
## 407 date 8 3.316612e-04
## 408 didn't 8 3.316612e-04
## 409 due 8 3.316612e-04
## 410 enough 8 3.316612e-04
## 411 everyone 8 3.316612e-04
## 412 generally 8 3.316612e-04
## 413 going 8 3.316612e-04
## 414 haven't 8 3.316612e-04
## 415 longer 8 3.316612e-04
## 416 lots 8 3.316612e-04
## 417 making 8 3.316612e-04
## 418 membership 8 3.316612e-04
## 419 mostly 8 3.316612e-04
## 420 numbers 8 3.316612e-04
## 421 option 8 3.316612e-04
## 422 own 8 3.316612e-04
## 423 past 8 3.316612e-04
## 424 profit 8 3.316612e-04
## 425 question 8 3.316612e-04
## 426 re 8 3.316612e-04
## 427 record 8 3.316612e-04
## 428 renewal 8 3.316612e-04
## 429 seating 8 3.316612e-04
## 430 setup 8 3.316612e-04
## 431 solid 8 3.316612e-04
## 432 sure 8 3.316612e-04
## 433 technology 8 3.316612e-04
## 434 thing 8 3.316612e-04
## 435 three 8 3.316612e-04
## 436 ticketagent 8 3.316612e-04
## 437 upgrades 8 3.316612e-04
## 438 users 8 3.316612e-04
## 439 we're 8 3.316612e-04
## 440 web 8 3.316612e-04
## 441 why 8 3.316612e-04
## 442 answers 7 2.902036e-04
## 443 awesome 7 2.902036e-04
## 444 complaints 7 2.902036e-04
## 445 consistent 7 2.902036e-04
## 446 continue 7 2.902036e-04
## 447 customization 7 2.902036e-04
## 448 donors 7 2.902036e-04
## 449 easily 7 2.902036e-04
## 450 error 7 2.902036e-04
## 451 example 7 2.902036e-04
## 452 except 7 2.902036e-04
## 453 far 7 2.902036e-04
## 454 financial 7 2.902036e-04
## 455 forward 7 2.902036e-04
## 456 got 7 2.902036e-04
## 457 growing 7 2.902036e-04
## 458 guys 7 2.902036e-04
## 459 improved 7 2.902036e-04
## 460 including 7 2.902036e-04
## 461 individual 7 2.902036e-04
## 462 info 7 2.902036e-04
## 463 isn't 7 2.902036e-04
## 464 learn 7 2.902036e-04
## 465 learning 7 2.902036e-04
## 466 level 7 2.902036e-04
## 467 limited 7 2.902036e-04
## 468 line 7 2.902036e-04
## 469 list 7 2.902036e-04
## 470 log 7 2.902036e-04
## 471 major 7 2.902036e-04
## 472 manager 7 2.902036e-04
## 473 might 7 2.902036e-04
## 474 months 7 2.902036e-04
## 475 old 7 2.902036e-04
## 476 please 7 2.902036e-04
## 477 positive 7 2.902036e-04
## 478 provide 7 2.902036e-04
## 479 provided 7 2.902036e-04
## 480 reasonable 7 2.902036e-04
## 481 reasons 7 2.902036e-04
## 482 recent 7 2.902036e-04
## 483 school 7 2.902036e-04
## 484 she 7 2.902036e-04
## 485 size 7 2.902036e-04
## 486 smaller 7 2.902036e-04
## 487 speak 7 2.902036e-04
## 488 these 7 2.902036e-04
## 489 training 7 2.902036e-04
## 490 trouble 7 2.902036e-04
## 491 type 7 2.902036e-04
## 492 update 7 2.902036e-04
## 493 updating 7 2.902036e-04
## 494 within 7 2.902036e-04
## 495 5 6 2.487459e-04
## 496 8 6 2.487459e-04
## 497 allow 6 2.487459e-04
## 498 although 6 2.487459e-04
## 499 amount 6 2.487459e-04
## 500 assistance 6 2.487459e-04
## 501 audience 6 2.487459e-04
## 502 capability 6 2.487459e-04
## 503 charge 6 2.487459e-04
## 504 click 6 2.487459e-04
## 505 communication 6 2.487459e-04
## 506 community 6 2.487459e-04
## 507 concerns 6 2.487459e-04
## 508 concert 6 2.487459e-04
## 509 constant 6 2.487459e-04
## 510 contract 6 2.487459e-04
## 511 costly 6 2.487459e-04
## 512 customize 6 2.487459e-04
## 513 difficulty 6 2.487459e-04
## 514 fit 6 2.487459e-04
## 515 frustrating 6 2.487459e-04
## 516 full 6 2.487459e-04
## 517 history 6 2.487459e-04
## 518 hours 6 2.487459e-04
## 519 income 6 2.487459e-04
## 520 market 6 2.487459e-04
## 521 means 6 2.487459e-04
## 522 media 6 2.487459e-04
## 523 member 6 2.487459e-04
## 524 operation 6 2.487459e-04
## 525 outstanding 6 2.487459e-04
## 526 performance 6 2.487459e-04
## 527 personal 6 2.487459e-04
## 528 place 6 2.487459e-04
## 529 point 6 2.487459e-04
## 530 powerful 6 2.487459e-04
## 531 printers 6 2.487459e-04
## 532 provides 6 2.487459e-04
## 533 purposes 6 2.487459e-04
## 534 quickly 6 2.487459e-04
## 535 real 6 2.487459e-04
## 536 requested 6 2.487459e-04
## 537 resolved 6 2.487459e-04
## 538 screen 6 2.487459e-04
## 539 security 6 2.487459e-04
## 540 send 6 2.487459e-04
## 541 sent 6 2.487459e-04
## 542 signed 6 2.487459e-04
## 543 social 6 2.487459e-04
## 544 systems 6 2.487459e-04
## 545 takes 6 2.487459e-04
## 546 thanks 6 2.487459e-04
## 547 that's 6 2.487459e-04
## 548 then 6 2.487459e-04
## 549 understand 6 2.487459e-04
## 550 via 6 2.487459e-04
## 551 waiting 6 2.487459e-04
## 552 we'd 6 2.487459e-04
## 553 week 6 2.487459e-04
## 554 whole 6 2.487459e-04
## 555 won't 6 2.487459e-04
## 556 6 5 2.072883e-04
## 557 7 5 2.072883e-04
## 558 9 5 2.072883e-04
## 559 absolutely 5 2.072883e-04
## 560 accounting 5 2.072883e-04
## 561 actually 5 2.072883e-04
## 562 address 5 2.072883e-04
## 563 android 5 2.072883e-04
## 564 appreciate 5 2.072883e-04
## 565 apps 5 2.072883e-04
## 566 area 5 2.072883e-04
## 567 aren't 5 2.072883e-04
## 568 ask 5 2.072883e-04
## 569 aspects 5 2.072883e-04
## 570 base 5 2.072883e-04
## 571 benefits 5 2.072883e-04
## 572 budget 5 2.072883e-04
## 573 bugs 5 2.072883e-04
## 574 building 5 2.072883e-04
## 575 button 5 2.072883e-04
## 576 buy 5 2.072883e-04
## 577 canadian 5 2.072883e-04
## 578 cards 5 2.072883e-04
## 579 charged 5 2.072883e-04
## 580 charges 5 2.072883e-04
## 581 cloud 5 2.072883e-04
## 582 compared 5 2.072883e-04
## 583 confusing 5 2.072883e-04
## 584 created 5 2.072883e-04
## 585 deal 5 2.072883e-04
## 586 designed 5 2.072883e-04
## 587 down 5 2.072883e-04
## 588 during 5 2.072883e-04
## 589 effective 5 2.072883e-04
## 590 efficient 5 2.072883e-04
## 591 else 5 2.072883e-04
## 592 entered 5 2.072883e-04
## 593 felt 5 2.072883e-04
## 594 finally 5 2.072883e-04
## 595 free 5 2.072883e-04
## 596 general 5 2.072883e-04
## 597 happen 5 2.072883e-04
## 598 hold 5 2.072883e-04
## 599 implemented 5 2.072883e-04
## 600 integration 5 2.072883e-04
## 601 items 5 2.072883e-04
## 602 keeps 5 2.072883e-04
## 603 let 5 2.072883e-04
## 604 link 5 2.072883e-04
## 605 main 5 2.072883e-04
## 606 manage 5 2.072883e-04
## 607 meets 5 2.072883e-04
## 608 memberships 5 2.072883e-04
## 609 numerous 5 2.072883e-04
## 610 offered 5 2.072883e-04
## 611 otherwise 5 2.072883e-04
## 612 package 5 2.072883e-04
## 613 page 5 2.072883e-04
## 614 pay 5 2.072883e-04
## 615 payment 5 2.072883e-04
## 616 plus 5 2.072883e-04
## 617 poor 5 2.072883e-04
## 618 potential 5 2.072883e-04
## 619 professionalism 5 2.072883e-04
## 620 promised 5 2.072883e-04
## 621 rather 5 2.072883e-04
## 622 reach 5 2.072883e-04
## 623 recently 5 2.072883e-04
## 624 records 5 2.072883e-04
## 625 recurring 5 2.072883e-04
## 626 relationship 5 2.072883e-04
## 627 respond 5 2.072883e-04
## 628 right 5 2.072883e-04
## 629 save 5 2.072883e-04
## 630 search 5 2.072883e-04
## 631 seen 5 2.072883e-04
## 632 separate 5 2.072883e-04
## 633 side 5 2.072883e-04
## 634 simplicity 5 2.072883e-04
## 635 smith 5 2.072883e-04
## 636 solutions 5 2.072883e-04
## 637 sort 5 2.072883e-04
## 638 start 5 2.072883e-04
## 639 started 5 2.072883e-04
## 640 structure 5 2.072883e-04
## 641 subscriptions 5 2.072883e-04
## 642 suggestions 5 2.072883e-04
## 643 tell 5 2.072883e-04
## 644 terms 5 2.072883e-04
## 645 theatres 5 2.072883e-04
## 646 thought 5 2.072883e-04
## 647 top 5 2.072883e-04
## 648 try 5 2.072883e-04
## 649 unable 5 2.072883e-04
## 650 under 5 2.072883e-04
## 651 usability 5 2.072883e-04
## 652 usually 5 2.072883e-04
## 653 vendor 5 2.072883e-04
## 654 accounts 4 1.658306e-04
## 655 accurate 4 1.658306e-04
## 656 additional 4 1.658306e-04
## 657 adult 4 1.658306e-04
## 658 afford 4 1.658306e-04
## 659 amounts 4 1.658306e-04
## 660 appropriate 4 1.658306e-04
## 661 attractive 4 1.658306e-04
## 662 availability 4 1.658306e-04
## 663 away 4 1.658306e-04
## 664 between 4 1.658306e-04
## 665 bigger 4 1.658306e-04
## 666 blasts 4 1.658306e-04
## 667 calendar 4 1.658306e-04
## 668 calls 4 1.658306e-04
## 669 certainly 4 1.658306e-04
## 670 check 4 1.658306e-04
## 671 class 4 1.658306e-04
## 672 clear 4 1.658306e-04
## 673 clunky 4 1.658306e-04
## 674 codes 4 1.658306e-04
## 675 colleagues 4 1.658306e-04
## 676 come 4 1.658306e-04
## 677 comments 4 1.658306e-04
## 678 competitors 4 1.658306e-04
## 679 complaint 4 1.658306e-04
## 680 complete 4 1.658306e-04
## 681 completely 4 1.658306e-04
## 682 complicated 4 1.658306e-04
## 683 comprehensive 4 1.658306e-04
## 684 control 4 1.658306e-04
## 685 corrected 4 1.658306e-04
## 686 cumbersome 4 1.658306e-04
## 687 current 4 1.658306e-04
## 688 custom 4 1.658306e-04
## 689 definitely 4 1.658306e-04
## 690 deposit 4 1.658306e-04
## 691 disappointed 4 1.658306e-04
## 692 dollars 4 1.658306e-04
## 693 enter 4 1.658306e-04
## 694 expected 4 1.658306e-04
## 695 fast 4 1.658306e-04
## 696 fits 4 1.658306e-04
## 697 fixes 4 1.658306e-04
## 698 focus 4 1.658306e-04
## 699 followed 4 1.658306e-04
## 700 four 4 1.658306e-04
## 701 giving 4 1.658306e-04
## 702 group 4 1.658306e-04
## 703 groups 4 1.658306e-04
## 704 hear 4 1.658306e-04
## 705 helped 4 1.658306e-04
## 706 here 4 1.658306e-04
## 707 ignored 4 1.658306e-04
## 708 improve 4 1.658306e-04
## 709 improvement 4 1.658306e-04
## 710 increased 4 1.658306e-04
## 711 initial 4 1.658306e-04
## 712 integrated 4 1.658306e-04
## 713 itself 4 1.658306e-04
## 714 job 4 1.658306e-04
## 715 kind 4 1.658306e-04
## 716 lacking 4 1.658306e-04
## 717 large 4 1.658306e-04
## 718 listen 4 1.658306e-04
## 719 lists 4 1.658306e-04
## 720 manually 4 1.658306e-04
## 721 message 4 1.658306e-04
## 722 met 4 1.658306e-04
## 723 monthly 4 1.658306e-04
## 724 nearly 4 1.658306e-04
## 725 nor 4 1.658306e-04
## 726 ok 4 1.658306e-04
## 727 once 4 1.658306e-04
## 728 operations 4 1.658306e-04
## 729 ours 4 1.658306e-04
## 730 pages 4 1.658306e-04
## 731 part 4 1.658306e-04
## 732 patron's 4 1.658306e-04
## 733 percentage 4 1.658306e-04
## 734 performances 4 1.658306e-04
## 735 pricey 4 1.658306e-04
## 736 products 4 1.658306e-04
## 737 programs 4 1.658306e-04
## 738 promises 4 1.658306e-04
## 739 pull 4 1.658306e-04
## 740 purchased 4 1.658306e-04
## 741 purchasing 4 1.658306e-04
## 742 quirks 4 1.658306e-04
## 743 quite 4 1.658306e-04
## 744 rate 4 1.658306e-04
## 745 regarding 4 1.658306e-04
## 746 rep 4 1.658306e-04
## 747 result 4 1.658306e-04
## 748 return 4 1.658306e-04
## 749 saying 4 1.658306e-04
## 750 second 4 1.658306e-04
## 751 section 4 1.658306e-04
## 752 select 4 1.658306e-04
## 753 series 4 1.658306e-04
## 754 serves 4 1.658306e-04
## 755 showing 4 1.658306e-04
## 756 simply 4 1.658306e-04
## 757 slightly 4 1.658306e-04
## 758 soon 4 1.658306e-04
## 759 sophisticated 4 1.658306e-04
## 760 special 4 1.658306e-04
## 761 specifically 4 1.658306e-04
## 762 starting 4 1.658306e-04
## 763 stay 4 1.658306e-04
## 764 staying 4 1.658306e-04
## 765 students 4 1.658306e-04
## 766 subscription 4 1.658306e-04
## 767 success 4 1.658306e-04
## 768 talk 4 1.658306e-04
## 769 took 4 1.658306e-04
## 770 transaction 4 1.658306e-04
## 771 tried 4 1.658306e-04
## 772 types 4 1.658306e-04
## 773 unhappy 4 1.658306e-04
## 774 until 4 1.658306e-04
## 775 utilize 4 1.658306e-04
## 776 valuable 4 1.658306e-04
## 777 value 4 1.658306e-04
## 778 venue 4 1.658306e-04
## 779 wait 4 1.658306e-04
## 780 wants 4 1.658306e-04
## 781 wrong 4 1.658306e-04
## 782 0 3 1.243730e-04
## 783 2012 3 1.243730e-04
## 784 24 3 1.243730e-04
## 785 30 3 1.243730e-04
## 786 33 3 1.243730e-04
## 787 abilities 3 1.243730e-04
## 788 actual 3 1.243730e-04
## 789 added 3 1.243730e-04
## 790 adding 3 1.243730e-04
## 791 addition 3 1.243730e-04
## 792 addressed 3 1.243730e-04
## 793 adults 3 1.243730e-04
## 794 advance 3 1.243730e-04
## 795 advantage 3 1.243730e-04
## 796 affordable 3 1.243730e-04
## 797 agents 3 1.243730e-04
## 798 allowed 3 1.243730e-04
## 799 allows 3 1.243730e-04
## 800 analysis 3 1.243730e-04
## 801 anyone 3 1.243730e-04
## 802 anywhere 3 1.243730e-04
## 803 appears 3 1.243730e-04
## 804 apple 3 1.243730e-04
## 805 application 3 1.243730e-04
## 806 apply 3 1.243730e-04
## 807 arounds 3 1.243730e-04
## 808 arts 3 1.243730e-04
## 809 bank 3 1.243730e-04
## 810 become 3 1.243730e-04
## 811 billing 3 1.243730e-04
## 812 breaks 3 1.243730e-04
## 813 bug 3 1.243730e-04
## 814 buggy 3 1.243730e-04
## 815 build 3 1.243730e-04
## 816 buttons 3 1.243730e-04
## 817 buyers 3 1.243730e-04
## 818 buying 3 1.243730e-04
## 819 calling 3 1.243730e-04
## 820 changed 3 1.243730e-04
## 821 chart 3 1.243730e-04
## 822 chip 3 1.243730e-04
## 823 choice 3 1.243730e-04
## 824 clearly 3 1.243730e-04
## 825 colleague 3 1.243730e-04
## 826 college 3 1.243730e-04
## 827 combined 3 1.243730e-04
## 828 coming 3 1.243730e-04
## 829 communications 3 1.243730e-04
## 830 compare 3 1.243730e-04
## 831 component 3 1.243730e-04
## 832 components 3 1.243730e-04
## 833 concern 3 1.243730e-04
## 834 confirmation 3 1.243730e-04
## 835 consider 3 1.243730e-04
## 836 continues 3 1.243730e-04
## 837 convenient 3 1.243730e-04
## 838 correct 3 1.243730e-04
## 839 correctly 3 1.243730e-04
## 840 costs 3 1.243730e-04
## 841 creating 3 1.243730e-04
## 842 creation 3 1.243730e-04
## 843 critical 3 1.243730e-04
## 844 curve 3 1.243730e-04
## 845 daily 3 1.243730e-04
## 846 de 3 1.243730e-04
## 847 dealing 3 1.243730e-04
## 848 delete 3 1.243730e-04
## 849 depending 3 1.243730e-04
## 850 desk 3 1.243730e-04
## 851 despite 3 1.243730e-04
## 852 detailed 3 1.243730e-04
## 853 direct 3 1.243730e-04
## 854 discounts 3 1.243730e-04
## 855 displayed 3 1.243730e-04
## 856 doing 3 1.243730e-04
## 857 dottie 3 1.243730e-04
## 858 engineering 3 1.243730e-04
## 859 english 3 1.243730e-04
## 860 enhancements 3 1.243730e-04
## 861 equipment 3 1.243730e-04
## 862 errors 3 1.243730e-04
## 863 exactly 3 1.243730e-04
## 864 exchange 3 1.243730e-04
## 865 exchanges 3 1.243730e-04
## 866 fault 3 1.243730e-04
## 867 feels 3 1.243730e-04
## 868 festival 3 1.243730e-04
## 869 fields 3 1.243730e-04
## 870 figure 3 1.243730e-04
## 871 flagged 3 1.243730e-04
## 872 florida 3 1.243730e-04
## 873 following 3 1.243730e-04
## 874 followup 3 1.243730e-04
## 875 form 3 1.243730e-04
## 876 format 3 1.243730e-04
## 877 front 3 1.243730e-04
## 878 frustrated 3 1.243730e-04
## 879 fundraising 3 1.243730e-04
## 880 further 3 1.243730e-04
## 881 future 3 1.243730e-04
## 882 gc 3 1.243730e-04
## 883 gets 3 1.243730e-04
## 884 glitchy 3 1.243730e-04
## 885 goes 3 1.243730e-04
## 886 google 3 1.243730e-04
## 887 growth 3 1.243730e-04
## 888 he 3 1.243730e-04
## 889 highly 3 1.243730e-04
## 890 hope 3 1.243730e-04
## 891 house 3 1.243730e-04
## 892 huge 3 1.243730e-04
## 893 hundreds 3 1.243730e-04
## 894 improving 3 1.243730e-04
## 895 inaccurate 3 1.243730e-04
## 896 inconsistent 3 1.243730e-04
## 897 increasing 3 1.243730e-04
## 898 integrate 3 1.243730e-04
## 899 internet 3 1.243730e-04
## 900 james 3 1.243730e-04
## 901 judy 3 1.243730e-04
## 902 keeping 3 1.243730e-04
## 903 key 3 1.243730e-04
## 904 knowledge 3 1.243730e-04
## 905 knows 3 1.243730e-04
## 906 later 3 1.243730e-04
## 907 least 3 1.243730e-04
## 908 left 3 1.243730e-04
## 909 letters 3 1.243730e-04
## 910 life 3 1.243730e-04
## 911 likely 3 1.243730e-04
## 912 limitation 3 1.243730e-04
## 913 lines 3 1.243730e-04
## 914 links 3 1.243730e-04
## 915 login 3 1.243730e-04
## 916 lost 3 1.243730e-04
## 917 low 3 1.243730e-04
## 918 manipulate 3 1.243730e-04
## 919 manner 3 1.243730e-04
## 920 maps 3 1.243730e-04
## 921 missing 3 1.243730e-04
## 922 module 3 1.243730e-04
## 923 monies 3 1.243730e-04
## 924 month 3 1.243730e-04
## 925 mrs 3 1.243730e-04
## 926 museum 3 1.243730e-04
## 927 notch 3 1.243730e-04
## 928 occasionally 3 1.243730e-04
## 929 operating 3 1.243730e-04
## 930 ordering 3 1.243730e-04
## 931 organized 3 1.243730e-04
## 932 outside 3 1.243730e-04
## 933 ownership 3 1.243730e-04
## 934 passes 3 1.243730e-04
## 935 paying 3 1.243730e-04
## 936 platforms 3 1.243730e-04
## 937 portion 3 1.243730e-04
## 938 presentation 3 1.243730e-04
## 939 press 3 1.243730e-04
## 940 previous 3 1.243730e-04
## 941 prices 3 1.243730e-04
## 942 printed 3 1.243730e-04
## 943 printing 3 1.243730e-04
## 944 processes 3 1.243730e-04
## 945 programming 3 1.243730e-04
## 946 prompt 3 1.243730e-04
## 947 ready 3 1.243730e-04
## 948 recognized 3 1.243730e-04
## 949 recommending 3 1.243730e-04
## 950 redeem 3 1.243730e-04
## 951 regards 3 1.243730e-04
## 952 related 3 1.243730e-04
## 953 remains 3 1.243730e-04
## 954 representative 3 1.243730e-04
## 955 request 3 1.243730e-04
## 956 require 3 1.243730e-04
## 957 required 3 1.243730e-04
## 958 reservations 3 1.243730e-04
## 959 resolution 3 1.243730e-04
## 960 resources 3 1.243730e-04
## 961 responsiveness 3 1.243730e-04
## 962 retention 3 1.243730e-04
## 963 revenue 3 1.243730e-04
## 964 road 3 1.243730e-04
## 965 ron 3 1.243730e-04
## 966 round 3 1.243730e-04
## 967 scale 3 1.243730e-04
## 968 scanners 3 1.243730e-04
## 969 sections 3 1.243730e-04
## 970 selecting 3 1.243730e-04
## 971 seniors 3 1.243730e-04
## 972 sense 3 1.243730e-04
## 973 significant 3 1.243730e-04
## 974 solve 3 1.243730e-04
## 975 spot 3 1.243730e-04
## 976 steps 3 1.243730e-04
## 977 store 3 1.243730e-04
## 978 straightforward 3 1.243730e-04
## 979 strong 3 1.243730e-04
## 980 student 3 1.243730e-04
## 981 subscribers 3 1.243730e-04
## 982 super 3 1.243730e-04
## 983 switch 3 1.243730e-04
## 984 symphony 3 1.243730e-04
## 985 taken 3 1.243730e-04
## 986 tasks 3 1.243730e-04
## 987 teach 3 1.243730e-04
## 988 technological 3 1.243730e-04
## 989 tedious 3 1.243730e-04
## 990 terrific 3 1.243730e-04
## 991 text 3 1.243730e-04
## 992 theaters 3 1.243730e-04
## 993 therefore 3 1.243730e-04
## 994 today 3 1.243730e-04
## 995 together 3 1.243730e-04
## 996 transactions 3 1.243730e-04
## 997 tries 3 1.243730e-04
## 998 trying 3 1.243730e-04
## 999 twice 3 1.243730e-04
## 1000 ui 3 1.243730e-04
## 1001 ultimately 3 1.243730e-04
## 1002 unless 3 1.243730e-04
## 1003 useless 3 1.243730e-04
## 1004 various 3 1.243730e-04
## 1005 vendini 3 1.243730e-04
## 1006 vendors 3 1.243730e-04
## 1007 versatility 3 1.243730e-04
## 1008 video 3 1.243730e-04
## 1009 view 3 1.243730e-04
## 1010 volunteers 3 1.243730e-04
## 1011 vs 3 1.243730e-04
## 1012 weeks 3 1.243730e-04
## 1013 weren't 3 1.243730e-04
## 1014 willing 3 1.243730e-04
## 1015 window 3 1.243730e-04
## 1016 windows 3 1.243730e-04
## 1017 worry 3 1.243730e-04
## 1018 wouldn't 3 1.243730e-04
## 1019 writer 3 1.243730e-04
## 1020 you're 3 1.243730e-04
## 1021 15 2 8.291530e-05
## 1022 200 2 8.291530e-05
## 1023 2011 2 8.291530e-05
## 1024 2016 2 8.291530e-05
## 1025 accessibility 2 8.291530e-05
## 1026 accessible 2 8.291530e-05
## 1027 accommodate 2 8.291530e-05
## 1028 action 2 8.291530e-05
## 1029 additionally 2 8.291530e-05
## 1030 addressing 2 8.291530e-05
## 1031 adequate 2 8.291530e-05
## 1032 administer 2 8.291530e-05
## 1033 advanced 2 8.291530e-05
## 1034 advances 2 8.291530e-05
## 1035 aid 2 8.291530e-05
## 1036 almost 2 8.291530e-05
## 1037 annoying 2 8.291530e-05
## 1038 answered 2 8.291530e-05
## 1039 archaic 2 8.291530e-05
## 1040 armed 2 8.291530e-05
## 1041 arrangements 2 8.291530e-05
## 1042 asked 2 8.291530e-05
## 1043 asking 2 8.291530e-05
## 1044 aspect 2 8.291530e-05
## 1045 assist 2 8.291530e-05
## 1046 associated 2 8.291530e-05
## 1047 attempted 2 8.291530e-05
## 1048 attendance 2 8.291530e-05
## 1049 automatic 2 8.291530e-05
## 1050 automatically 2 8.291530e-05
## 1051 bad 2 8.291530e-05
## 1052 balance 2 8.291530e-05
## 1053 bar 2 8.291530e-05
## 1054 basically 2 8.291530e-05
## 1055 basis 2 8.291530e-05
## 1056 basket 2 8.291530e-05
## 1057 became 2 8.291530e-05
## 1058 beginning 2 8.291530e-05
## 1059 behalf 2 8.291530e-05
## 1060 behind 2 8.291530e-05
## 1061 believe 2 8.291530e-05
## 1062 below 2 8.291530e-05
## 1063 benefit 2 8.291530e-05
## 1064 big 2 8.291530e-05
## 1065 biggest 2 8.291530e-05
## 1066 boca 2 8.291530e-05
## 1067 book 2 8.291530e-05
## 1068 botched 2 8.291530e-05
## 1069 breach 2 8.291530e-05
## 1070 bring 2 8.291530e-05
## 1071 brings 2 8.291530e-05
## 1072 broken 2 8.291530e-05
## 1073 browsers 2 8.291530e-05
## 1074 builder 2 8.291530e-05
## 1075 built 2 8.291530e-05
## 1076 bunch 2 8.291530e-05
## 1077 buyer 2 8.291530e-05
## 1078 cableone 2 8.291530e-05
## 1079 came 2 8.291530e-05
## 1080 case 2 8.291530e-05
## 1081 caused 2 8.291530e-05
## 1082 central 2 8.291530e-05
## 1083 challenged 2 8.291530e-05
## 1084 challenging 2 8.291530e-05
## 1085 changing 2 8.291530e-05
## 1086 charts 2 8.291530e-05
## 1087 cheaper 2 8.291530e-05
## 1088 cheyenne 2 8.291530e-05
## 1089 choosing 2 8.291530e-05
## 1090 close 2 8.291530e-05
## 1091 coast 2 8.291530e-05
## 1092 collect 2 8.291530e-05
## 1093 collected 2 8.291530e-05
## 1094 colors 2 8.291530e-05
## 1095 combination 2 8.291530e-05
## 1096 combine 2 8.291530e-05
## 1097 comes 2 8.291530e-05
## 1098 comfortable 2 8.291530e-05
## 1099 common 2 8.291530e-05
## 1100 communicating 2 8.291530e-05
## 1101 comparable 2 8.291530e-05
## 1102 competent 2 8.291530e-05
## 1103 competitive 2 8.291530e-05
## 1104 completed 2 8.291530e-05
## 1105 complex 2 8.291530e-05
## 1106 compromised 2 8.291530e-05
## 1107 concerned 2 8.291530e-05
## 1108 concise 2 8.291530e-05
## 1109 considered 2 8.291530e-05
## 1110 consistently 2 8.291530e-05
## 1111 consuming 2 8.291530e-05
## 1112 contacted 2 8.291530e-05
## 1113 continued 2 8.291530e-05
## 1114 conversation 2 8.291530e-05
## 1115 costumer 2 8.291530e-05
## 1116 couldn't 2 8.291530e-05
## 1117 couple 2 8.291530e-05
## 1118 courteous 2 8.291530e-05
## 1119 customizable 2 8.291530e-05
## 1120 customized 2 8.291530e-05
## 1121 declined 2 8.291530e-05
## 1122 delay 2 8.291530e-05
## 1123 deleting 2 8.291530e-05
## 1124 demographics 2 8.291530e-05
## 1125 departments 2 8.291530e-05
## 1126 depend 2 8.291530e-05
## 1127 dependable 2 8.291530e-05
## 1128 depends 2 8.291530e-05
## 1129 desktop 2 8.291530e-05
## 1130 develop 2 8.291530e-05
## 1131 device 2 8.291530e-05
## 1132 directly 2 8.291530e-05
## 1133 director 2 8.291530e-05
## 1134 directors 2 8.291530e-05
## 1135 disappointing 2 8.291530e-05
## 1136 documents 2 8.291530e-05
## 1137 door 2 8.291530e-05
## 1138 dropped 2 8.291530e-05
## 1139 dynamic 2 8.291530e-05
## 1140 eager 2 8.291530e-05
## 1141 easiest 2 8.291530e-05
## 1142 edit 2 8.291530e-05
## 1143 editing 2 8.291530e-05
## 1144 effort 2 8.291530e-05
## 1145 efforts 2 8.291530e-05
## 1146 either 2 8.291530e-05
## 1147 elsewhere 2 8.291530e-05
## 1148 emailed 2 8.291530e-05
## 1149 emailing 2 8.291530e-05
## 1150 enjoy 2 8.291530e-05
## 1151 entry 2 8.291530e-05
## 1152 escape 2 8.291530e-05
## 1153 evening 2 8.291530e-05
## 1154 eventbrite 2 8.291530e-05
## 1155 exist 2 8.291530e-05
## 1156 expense 2 8.291530e-05
## 1157 exploring 2 8.291530e-05
## 1158 exported 2 8.291530e-05
## 1159 extra 2 8.291530e-05
## 1160 extract 2 8.291530e-05
## 1161 fabulous 2 8.291530e-05
## 1162 facilitate 2 8.291530e-05
## 1163 failure 2 8.291530e-05
## 1164 fair 2 8.291530e-05
## 1165 fall 2 8.291530e-05
## 1166 familiar 2 8.291530e-05
## 1167 faster 2 8.291530e-05
## 1168 feedback 2 8.291530e-05
## 1169 festivals 2 8.291530e-05
## 1170 files 2 8.291530e-05
## 1171 finding 2 8.291530e-05
## 1172 fine 2 8.291530e-05
## 1173 flash 2 8.291530e-05
## 1174 flawed 2 8.291530e-05
## 1175 flaws 2 8.291530e-05
## 1176 focused 2 8.291530e-05
## 1177 forced 2 8.291530e-05
## 1178 forces 2 8.291530e-05
## 1179 formatting 2 8.291530e-05
## 1180 frequently 2 8.291530e-05
## 1181 friend 2 8.291530e-05
## 1182 friends 2 8.291530e-05
## 1183 functional 2 8.291530e-05
## 1184 generation 2 8.291530e-05
## 1185 gift 2 8.291530e-05
## 1186 gone 2 8.291530e-05
## 1187 graphics 2 8.291530e-05
## 1188 greater 2 8.291530e-05
## 1189 grow 2 8.291530e-05
## 1190 guest 2 8.291530e-05
## 1191 handled 2 8.291530e-05
## 1192 happened 2 8.291530e-05
## 1193 hardware 2 8.291530e-05
## 1194 heard 2 8.291530e-05
## 1195 helping 2 8.291530e-05
## 1196 her 2 8.291530e-05
## 1197 holders 2 8.291530e-05
## 1198 holds 2 8.291530e-05
## 1199 home 2 8.291530e-05
## 1200 hunter 2 8.291530e-05
## 1201 husband 2 8.291530e-05
## 1202 i.e 2 8.291530e-05
## 1203 ideal 2 8.291530e-05
## 1204 identified 2 8.291530e-05
## 1205 images 2 8.291530e-05
## 1206 immediately 2 8.291530e-05
## 1207 impacted 2 8.291530e-05
## 1208 implement 2 8.291530e-05
## 1209 implementation 2 8.291530e-05
## 1210 implementing 2 8.291530e-05
## 1211 impossible 2 8.291530e-05
## 1212 impression 2 8.291530e-05
## 1213 inconvenient 2 8.291530e-05
## 1214 increase 2 8.291530e-05
## 1215 innovation 2 8.291530e-05
## 1216 input 2 8.291530e-05
## 1217 inquiries 2 8.291530e-05
## 1218 instance 2 8.291530e-05
## 1219 integrations 2 8.291530e-05
## 1220 integrity 2 8.291530e-05
## 1221 interact 2 8.291530e-05
## 1222 interested 2 8.291530e-05
## 1223 interfaces 2 8.291530e-05
## 1224 inventory 2 8.291530e-05
## 1225 investigate 2 8.291530e-05
## 1226 jar 2 8.291530e-05
## 1227 kicks 2 8.291530e-05
## 1228 kids 2 8.291530e-05
## 1229 knowledgeable 2 8.291530e-05
## 1230 l 2 8.291530e-05
## 1231 laborious 2 8.291530e-05
## 1232 lacks 2 8.291530e-05
## 1233 legal 2 8.291530e-05
## 1234 letter 2 8.291530e-05
## 1235 live 2 8.291530e-05
## 1236 load 2 8.291530e-05
## 1237 loading 2 8.291530e-05
## 1238 locations 2 8.291530e-05
## 1239 looks 2 8.291530e-05
## 1240 lower 2 8.291530e-05
## 1241 lowest 2 8.291530e-05
## 1242 mailchimp 2 8.291530e-05
## 1243 mailing 2 8.291530e-05
## 1244 mailings 2 8.291530e-05
## 1245 mails 2 8.291530e-05
## 1246 mainly 2 8.291530e-05
## 1247 majority 2 8.291530e-05
## 1248 managing 2 8.291530e-05
## 1249 manual 2 8.291530e-05
## 1250 mary 2 8.291530e-05
## 1251 mass 2 8.291530e-05
## 1252 match 2 8.291530e-05
## 1253 maybe 2 8.291530e-05
## 1254 meet 2 8.291530e-05
## 1255 middle 2 8.291530e-05
## 1256 minutes 2 8.291530e-05
## 1257 monitor 2 8.291530e-05
## 1258 move 2 8.291530e-05
## 1259 moving 2 8.291530e-05
## 1260 mr 2 8.291530e-05
## 1261 myself 2 8.291530e-05
## 1262 n 2 8.291530e-05
## 1263 names 2 8.291530e-05
## 1264 nature 2 8.291530e-05
## 1265 needing 2 8.291530e-05
## 1266 net 2 8.291530e-05
## 1267 newsletter 2 8.291530e-05
## 1268 night 2 8.291530e-05
## 1269 nightmare 2 8.291530e-05
## 1270 obviously 2 8.291530e-05
## 1271 oddities 2 8.291530e-05
## 1272 offices 2 8.291530e-05
## 1273 oh 2 8.291530e-05
## 1274 older 2 8.291530e-05
## 1275 ones 2 8.291530e-05
## 1276 onto 2 8.291530e-05
## 1277 open 2 8.291530e-05
## 1278 operator 2 8.291530e-05
## 1279 opinion 2 8.291530e-05
## 1280 organizational 2 8.291530e-05
## 1281 orgs 2 8.291530e-05
## 1282 os 2 8.291530e-05
## 1283 output 2 8.291530e-05
## 1284 pace 2 8.291530e-05
## 1285 packaging 2 8.291530e-05
## 1286 particular 2 8.291530e-05
## 1287 particularly 2 8.291530e-05
## 1288 payments 2 8.291530e-05
## 1289 pc 2 8.291530e-05
## 1290 pending 2 8.291530e-05
## 1291 perform 2 8.291530e-05
## 1292 perhaps 2 8.291530e-05
## 1293 permanent 2 8.291530e-05
## 1294 phoenix 2 8.291530e-05
## 1295 plan 2 8.291530e-05
## 1296 play 2 8.291530e-05
## 1297 players 2 8.291530e-05
## 1298 political 2 8.291530e-05
## 1299 possible 2 8.291530e-05
## 1300 post 2 8.291530e-05
## 1301 posting 2 8.291530e-05
## 1302 prefer 2 8.291530e-05
## 1303 present 2 8.291530e-05
## 1304 presently 2 8.291530e-05
## 1305 priced 2 8.291530e-05
## 1306 primarily 2 8.291530e-05
## 1307 prior 2 8.291530e-05
## 1308 priority 2 8.291530e-05
## 1309 probably 2 8.291530e-05
## 1310 problematic 2 8.291530e-05
## 1311 procedures 2 8.291530e-05
## 1312 processor 2 8.291530e-05
## 1313 production 2 8.291530e-05
## 1314 productions 2 8.291530e-05
## 1315 productivity 2 8.291530e-05
## 1316 prohibitive 2 8.291530e-05
## 1317 promote 2 8.291530e-05
## 1318 properly 2 8.291530e-05
## 1319 providers 2 8.291530e-05
## 1320 providing 2 8.291530e-05
## 1321 pulling 2 8.291530e-05
## 1322 purpose 2 8.291530e-05
## 1323 push 2 8.291530e-05
## 1324 range 2 8.291530e-05
## 1325 rarely 2 8.291530e-05
## 1326 rating 2 8.291530e-05
## 1327 reader 2 8.291530e-05
## 1328 realize 2 8.291530e-05
## 1329 realized 2 8.291530e-05
## 1330 reasonably 2 8.291530e-05
## 1331 recommendation 2 8.291530e-05
## 1332 reconcile 2 8.291530e-05
## 1333 reconciling 2 8.291530e-05
## 1334 reflect 2 8.291530e-05
## 1335 refunds 2 8.291530e-05
## 1336 register 2 8.291530e-05
## 1337 regularly 2 8.291530e-05
## 1338 relationships 2 8.291530e-05
## 1339 relatively 2 8.291530e-05
## 1340 released 2 8.291530e-05
## 1341 remedy 2 8.291530e-05
## 1342 renew 2 8.291530e-05
## 1343 renewals 2 8.291530e-05
## 1344 repeated 2 8.291530e-05
## 1345 replies 2 8.291530e-05
## 1346 reported 2 8.291530e-05
## 1347 reps 2 8.291530e-05
## 1348 resolve 2 8.291530e-05
## 1349 resource 2 8.291530e-05
## 1350 respects 2 8.291530e-05
## 1351 resulting 2 8.291530e-05
## 1352 results 2 8.291530e-05
## 1353 rich 2 8.291530e-05
## 1354 rocks 2 8.291530e-05
## 1355 room 2 8.291530e-05
## 1356 rude 2 8.291530e-05
## 1357 salutation 2 8.291530e-05
## 1358 scanned 2 8.291530e-05
## 1359 scanner 2 8.291530e-05
## 1360 schedule 2 8.291530e-05
## 1361 scope 2 8.291530e-05
## 1362 seamless 2 8.291530e-05
## 1363 seasons 2 8.291530e-05
## 1364 secure 2 8.291530e-05
## 1365 seemingly 2 8.291530e-05
## 1366 selected 2 8.291530e-05
## 1367 self 2 8.291530e-05
## 1368 sending 2 8.291530e-05
## 1369 serious 2 8.291530e-05
## 1370 sessions 2 8.291530e-05
## 1371 sets 2 8.291530e-05
## 1372 sign 2 8.291530e-05
## 1373 significantly 2 8.291530e-05
## 1374 similar 2 8.291530e-05
## 1375 six 2 8.291530e-05
## 1376 specific 2 8.291530e-05
## 1377 spend 2 8.291530e-05
## 1378 stable 2 8.291530e-05
## 1379 standard 2 8.291530e-05
## 1380 stating 2 8.291530e-05
## 1381 status 2 8.291530e-05
## 1382 stop 2 8.291530e-05
## 1383 strongly 2 8.291530e-05
## 1384 submit 2 8.291530e-05
## 1385 subscriber 2 8.291530e-05
## 1386 summer 2 8.291530e-05
## 1387 superb 2 8.291530e-05
## 1388 supposed 2 8.291530e-05
## 1389 survey 2 8.291530e-05
## 1390 swipe 2 8.291530e-05
## 1391 t 2 8.291530e-05
## 1392 taking 2 8.291530e-05
## 1393 talked 2 8.291530e-05
## 1394 templates 2 8.291530e-05
## 1395 ten 2 8.291530e-05
## 1396 tend 2 8.291530e-05
## 1397 they're 2 8.291530e-05
## 1398 third 2 8.291530e-05
## 1399 thus 2 8.291530e-05
## 1400 ticketline 2 8.291530e-05
## 1401 tie 2 8.291530e-05
## 1402 tiny 2 8.291530e-05
## 1403 tip 2 8.291530e-05
## 1404 tix 2 8.291530e-05
## 1405 total 2 8.291530e-05
## 1406 train 2 8.291530e-05
## 1407 treasurer 2 8.291530e-05
## 1408 truly 2 8.291530e-05
## 1409 turnover 2 8.291530e-05
## 1410 understandable 2 8.291530e-05
## 1411 uninformed 2 8.291530e-05
## 1412 university 2 8.291530e-05
## 1413 unnecessary 2 8.291530e-05
## 1414 unresolved 2 8.291530e-05
## 1415 unsubscribe 2 8.291530e-05
## 1416 upgrade 2 8.291530e-05
## 1417 upload 2 8.291530e-05
## 1418 upset 2 8.291530e-05
## 1419 usable 2 8.291530e-05
## 1420 uses 2 8.291530e-05
## 1421 uso 2 8.291530e-05
## 1422 variety 2 8.291530e-05
## 1423 vending 2 8.291530e-05
## 1424 version 2 8.291530e-05
## 1425 vital 2 8.291530e-05
## 1426 wanting 2 8.291530e-05
## 1427 weak 2 8.291530e-05
## 1428 weekly 2 8.291530e-05
## 1429 went 2 8.291530e-05
## 1430 whatever 2 8.291530e-05
## 1431 whenever 2 8.291530e-05
## 1432 whether 2 8.291530e-05
## 1433 willingness 2 8.291530e-05
## 1434 wishes 2 8.291530e-05
## 1435 word 2 8.291530e-05
## 1436 workarounds 2 8.291530e-05
## 1437 workers 2 8.291530e-05
## 1438 worth 2 8.291530e-05
## 1439 writing 2 8.291530e-05
## 1440 yes 2 8.291530e-05
## 1441 zero 2 8.291530e-05
## 1442 10,000 1 4.145765e-05
## 1443 1000 1 4.145765e-05
## 1444 11 1 4.145765e-05
## 1445 12 1 4.145765e-05
## 1446 12month 1 4.145765e-05
## 1447 133334 1 4.145765e-05
## 1448 135 1 4.145765e-05
## 1449 16 1 4.145765e-05
## 1450 19.00 1 4.145765e-05
## 1451 20 1 4.145765e-05
## 1452 2004 1 4.145765e-05
## 1453 2008 1 4.145765e-05
## 1454 200dpi 1 4.145765e-05
## 1455 2015 1 4.145765e-05
## 1456 2017 1 4.145765e-05
## 1457 21 1 4.145765e-05
## 1458 3,000 1 4.145765e-05
## 1459 30,000 1 4.145765e-05
## 1460 30percent 1 4.145765e-05
## 1461 35 1 4.145765e-05
## 1462 36 1 4.145765e-05
## 1463 360 1 4.145765e-05
## 1464 3915 1 4.145765e-05
## 1465 475 1 4.145765e-05
## 1466 4th 1 4.145765e-05
## 1467 503 1 4.145765e-05
## 1468 594 1 4.145765e-05
## 1469 600 1 4.145765e-05
## 1470 80 1 4.145765e-05
## 1471 90 1 4.145765e-05
## 1472 95 1 4.145765e-05
## 1473 99 1 4.145765e-05
## 1474 ___ 1 4.145765e-05
## 1475 abillty 1 4.145765e-05
## 1476 acceptable 1 4.145765e-05
## 1477 accessing 1 4.145765e-05
## 1478 accommodating 1 4.145765e-05
## 1479 accomplished 1 4.145765e-05
## 1480 accountant 1 4.145765e-05
## 1481 accounted 1 4.145765e-05
## 1482 accuracy 1 4.145765e-05
## 1483 accustomed 1 4.145765e-05
## 1484 achievement 1 4.145765e-05
## 1485 across 1 4.145765e-05
## 1486 activating 1 4.145765e-05
## 1487 activity 1 4.145765e-05
## 1488 ad 1 4.145765e-05
## 1489 adjust 1 4.145765e-05
## 1490 adjustability 1 4.145765e-05
## 1491 adjusted 1 4.145765e-05
## 1492 adjustment 1 4.145765e-05
## 1493 admin 1 4.145765e-05
## 1494 administered 1 4.145765e-05
## 1495 administrator 1 4.145765e-05
## 1496 admission 1 4.145765e-05
## 1497 adore 1 4.145765e-05
## 1498 advertised 1 4.145765e-05
## 1499 advice 1 4.145765e-05
## 1500 affected 1 4.145765e-05
## 1501 affordability 1 4.145765e-05
## 1502 age 1 4.145765e-05
## 1503 agencies 1 4.145765e-05
## 1504 aggregate 1 4.145765e-05
## 1505 agreement 1 4.145765e-05
## 1506 ahead 1 4.145765e-05
## 1507 alert 1 4.145765e-05
## 1508 aligned 1 4.145765e-05
## 1509 alike 1 4.145765e-05
## 1510 alleviate 1 4.145765e-05
## 1511 allowing 1 4.145765e-05
## 1512 alot 1 4.145765e-05
## 1513 alphabetized 1 4.145765e-05
## 1514 altered 1 4.145765e-05
## 1515 among 1 4.145765e-05
## 1516 analytics 1 4.145765e-05
## 1517 announcements 1 4.145765e-05
## 1518 annoyance 1 4.145765e-05
## 1519 annual 1 4.145765e-05
## 1520 answering 1 4.145765e-05
## 1521 api 1 4.145765e-05
## 1522 apis 1 4.145765e-05
## 1523 apparently 1 4.145765e-05
## 1524 appear 1 4.145765e-05
## 1525 appearing 1 4.145765e-05
## 1526 applying 1 4.145765e-05
## 1527 appreciated 1 4.145765e-05
## 1528 appreciation 1 4.145765e-05
## 1529 appreciative 1 4.145765e-05
## 1530 approach 1 4.145765e-05
## 1531 approx 1 4.145765e-05
## 1532 arise 1 4.145765e-05
## 1533 arises 1 4.145765e-05
## 1534 arranged 1 4.145765e-05
## 1535 arranging 1 4.145765e-05
## 1536 array 1 4.145765e-05
## 1537 arrives 1 4.145765e-05
## 1538 ascertained 1 4.145765e-05
## 1539 aside 1 4.145765e-05
## 1540 ass 1 4.145765e-05
## 1541 asset 1 4.145765e-05
## 1542 assisting 1 4.145765e-05
## 1543 assured 1 4.145765e-05
## 1544 astounding 1 4.145765e-05
## 1545 aswering 1 4.145765e-05
## 1546 attempting 1 4.145765e-05
## 1547 attempts 1 4.145765e-05
## 1548 attend 1 4.145765e-05
## 1549 attending 1 4.145765e-05
## 1550 attention 1 4.145765e-05
## 1551 attentive 1 4.145765e-05
## 1552 attitude 1 4.145765e-05
## 1553 attitudes 1 4.145765e-05
## 1554 audiences 1 4.145765e-05
## 1555 august 1 4.145765e-05
## 1556 authorize 1 4.145765e-05
## 1557 auto 1 4.145765e-05
## 1558 automate 1 4.145765e-05
## 1559 automated 1 4.145765e-05
## 1560 average 1 4.145765e-05
## 1561 averrage 1 4.145765e-05
## 1562 aware 1 4.145765e-05
## 1563 awesomeness 1 4.145765e-05
## 1564 awful 1 4.145765e-05
## 1565 awkward 1 4.145765e-05
## 1566 b 1 4.145765e-05
## 1567 b2b 1 4.145765e-05
## 1568 backend 1 4.145765e-05
## 1569 backs 1 4.145765e-05
## 1570 baker 1 4.145765e-05
## 1571 ballet 1 4.145765e-05
## 1572 banking 1 4.145765e-05
## 1573 bare 1 4.145765e-05
## 1574 baseball 1 4.145765e-05
## 1575 bases 1 4.145765e-05
## 1576 basics 1 4.145765e-05
## 1577 batch 1 4.145765e-05
## 1578 bathrooms 1 4.145765e-05
## 1579 bay 1 4.145765e-05
## 1580 beautiful 1 4.145765e-05
## 1581 began 1 4.145765e-05
## 1582 behave 1 4.145765e-05
## 1583 bells 1 4.145765e-05
## 1584 betsy 1 4.145765e-05
## 1585 beyond 1 4.145765e-05
## 1586 billed 1 4.145765e-05
## 1587 bizarrely 1 4.145765e-05
## 1588 blamed 1 4.145765e-05
## 1589 blank 1 4.145765e-05
## 1590 blend 1 4.145765e-05
## 1591 blocked 1 4.145765e-05
## 1592 blocks 1 4.145765e-05
## 1593 blog 1 4.145765e-05
## 1594 board 1 4.145765e-05
## 1595 boasted 1 4.145765e-05
## 1596 bocca 1 4.145765e-05
## 1597 bolts 1 4.145765e-05
## 1598 bones 1 4.145765e-05
## 1599 bookkeeping 1 4.145765e-05
## 1600 bothered 1 4.145765e-05
## 1601 bouncing 1 4.145765e-05
## 1602 breadth 1 4.145765e-05
## 1603 breakdowns 1 4.145765e-05
## 1604 breech 1 4.145765e-05
## 1605 brief 1 4.145765e-05
## 1606 brilliant 1 4.145765e-05
## 1607 broad 1 4.145765e-05
## 1608 broadway 1 4.145765e-05
## 1609 browser 1 4.145765e-05
## 1610 bsc.edu 1 4.145765e-05
## 1611 bsctheatre 1 4.145765e-05
## 1612 bugginess 1 4.145765e-05
## 1613 bull 1 4.145765e-05
## 1614 bummer 1 4.145765e-05
## 1615 businesses 1 4.145765e-05
## 1616 buys 1 4.145765e-05
## 1617 bypass 1 4.145765e-05
## 1618 c 1 4.145765e-05
## 1619 calculate 1 4.145765e-05
## 1620 called 1 4.145765e-05
## 1621 camel's 1 4.145765e-05
## 1622 camp 1 4.145765e-05
## 1623 campaigns 1 4.145765e-05
## 1624 camper 1 4.145765e-05
## 1625 campus 1 4.145765e-05
## 1626 canada 1 4.145765e-05
## 1627 cancelled 1 4.145765e-05
## 1628 careful 1 4.145765e-05
## 1629 carefully 1 4.145765e-05
## 1630 carmel 1 4.145765e-05
## 1631 cases 1 4.145765e-05
## 1632 cash 1 4.145765e-05
## 1633 cashiers 1 4.145765e-05
## 1634 category 1 4.145765e-05
## 1635 cause 1 4.145765e-05
## 1636 causes 1 4.145765e-05
## 1637 causing 1 4.145765e-05
## 1638 caution 1 4.145765e-05
## 1639 celeste 1 4.145765e-05
## 1640 center 1 4.145765e-05
## 1641 century 1 4.145765e-05
## 1642 chair 1 4.145765e-05
## 1643 challenge 1 4.145765e-05
## 1644 challenges 1 4.145765e-05
## 1645 chamber 1 4.145765e-05
## 1646 chance 1 4.145765e-05
## 1647 charitable 1 4.145765e-05
## 1648 chat 1 4.145765e-05
## 1649 cheap 1 4.145765e-05
## 1650 cheating 1 4.145765e-05
## 1651 chief 1 4.145765e-05
## 1652 chimp 1 4.145765e-05
## 1653 choose 1 4.145765e-05
## 1654 chooses 1 4.145765e-05
## 1655 chrome 1 4.145765e-05
## 1656 city 1 4.145765e-05
## 1657 clackamas 1 4.145765e-05
## 1658 claiming 1 4.145765e-05
## 1659 clarity 1 4.145765e-05
## 1660 classes 1 4.145765e-05
## 1661 clicks 1 4.145765e-05
## 1662 clientele 1 4.145765e-05
## 1663 clumsy 1 4.145765e-05
## 1664 clutter 1 4.145765e-05
## 1665 cohesive 1 4.145765e-05
## 1666 collecting 1 4.145765e-05
## 1667 collection 1 4.145765e-05
## 1668 commitment 1 4.145765e-05
## 1669 committed 1 4.145765e-05
## 1670 communicate 1 4.145765e-05
## 1671 communicated 1 4.145765e-05
## 1672 comp 1 4.145765e-05
## 1673 company's 1 4.145765e-05
## 1674 comparing 1 4.145765e-05
## 1675 compatibility 1 4.145765e-05
## 1676 compatible 1 4.145765e-05
## 1677 compete 1 4.145765e-05
## 1678 competition 1 4.145765e-05
## 1679 complacency 1 4.145765e-05
## 1680 complain 1 4.145765e-05
## 1681 complained 1 4.145765e-05
## 1682 complains 1 4.145765e-05
## 1683 complexity 1 4.145765e-05
## 1684 compliance 1 4.145765e-05
## 1685 compliant 1 4.145765e-05
## 1686 composing 1 4.145765e-05
## 1687 comprehensiveness 1 4.145765e-05
## 1688 comps 1 4.145765e-05
## 1689 computers 1 4.145765e-05
## 1690 concerts 1 4.145765e-05
## 1691 concludes 1 4.145765e-05
## 1692 condescending 1 4.145765e-05
## 1693 conduct 1 4.145765e-05
## 1694 conference 1 4.145765e-05
## 1695 confusion 1 4.145765e-05
## 1696 connect 1 4.145765e-05
## 1697 connectibility 1 4.145765e-05
## 1698 connecting 1 4.145765e-05
## 1699 connections 1 4.145765e-05
## 1700 connectivity 1 4.145765e-05
## 1701 conscientious 1 4.145765e-05
## 1702 conscious 1 4.145765e-05
## 1703 consecutive 1 4.145765e-05
## 1704 conservative 1 4.145765e-05
## 1705 consideration 1 4.145765e-05
## 1706 consistency 1 4.145765e-05
## 1707 constantcontact 1 4.145765e-05
## 1708 consumer 1 4.145765e-05
## 1709 contacting 1 4.145765e-05
## 1710 contacts 1 4.145765e-05
## 1711 contain 1 4.145765e-05
## 1712 continuing 1 4.145765e-05
## 1713 continuous 1 4.145765e-05
## 1714 continuously 1 4.145765e-05
## 1715 contradict 1 4.145765e-05
## 1716 conv 1 4.145765e-05
## 1717 convoluted 1 4.145765e-05
## 1718 cookies 1 4.145765e-05
## 1719 copy 1 4.145765e-05
## 1720 corporate 1 4.145765e-05
## 1721 corporation 1 4.145765e-05
## 1722 costing 1 4.145765e-05
## 1723 costumers 1 4.145765e-05
## 1724 coupons 1 4.145765e-05
## 1725 cover 1 4.145765e-05
## 1726 covers 1 4.145765e-05
## 1727 cracks 1 4.145765e-05
## 1728 crap 1 4.145765e-05
## 1729 crash 1 4.145765e-05
## 1730 crazy 1 4.145765e-05
## 1731 creates 1 4.145765e-05
## 1732 crippling 1 4.145765e-05
## 1733 crude 1 4.145765e-05
## 1734 currently 1 4.145765e-05
## 1735 custermer 1 4.145765e-05
## 1736 customer's 1 4.145765e-05
## 1737 cuts 1 4.145765e-05
## 1738 cutting 1 4.145765e-05
## 1739 dance 1 4.145765e-05
## 1740 dare 1 4.145765e-05
## 1741 dashboard 1 4.145765e-05
## 1742 databases 1 4.145765e-05
## 1743 datas 1 4.145765e-05
## 1744 dated 1 4.145765e-05
## 1745 dates 1 4.145765e-05
## 1746 days 1 4.145765e-05
## 1747 dc 1 4.145765e-05
## 1748 debited 1 4.145765e-05
## 1749 decent 1 4.145765e-05
## 1750 decided 1 4.145765e-05
## 1751 decision 1 4.145765e-05
## 1752 decrease 1 4.145765e-05
## 1753 deducted 1 4.145765e-05
## 1754 deduction 1 4.145765e-05
## 1755 default 1 4.145765e-05
## 1756 deficiencies 1 4.145765e-05
## 1757 delays 1 4.145765e-05
## 1758 deliver 1 4.145765e-05
## 1759 delivers 1 4.145765e-05
## 1760 demands 1 4.145765e-05
## 1761 demographic 1 4.145765e-05
## 1762 demos 1 4.145765e-05
## 1763 department 1 4.145765e-05
## 1764 dependability 1 4.145765e-05
## 1765 deposits 1 4.145765e-05
## 1766 description 1 4.145765e-05
## 1767 designing 1 4.145765e-05
## 1768 desired 1 4.145765e-05
## 1769 desktops 1 4.145765e-05
## 1770 details 1 4.145765e-05
## 1771 determining 1 4.145765e-05
## 1772 developed 1 4.145765e-05
## 1773 deviations 1 4.145765e-05
## 1774 devices 1 4.145765e-05
## 1775 diane 1 4.145765e-05
## 1776 difficulties 1 4.145765e-05
## 1777 digitizes 1 4.145765e-05
## 1778 dinged 1 4.145765e-05
## 1779 dinners 1 4.145765e-05
## 1780 direction 1 4.145765e-05
## 1781 disappeared 1 4.145765e-05
## 1782 disbursement 1 4.145765e-05
## 1783 discounting 1 4.145765e-05
## 1784 discover 1 4.145765e-05
## 1785 discuss 1 4.145765e-05
## 1786 disdain 1 4.145765e-05
## 1787 disengage 1 4.145765e-05
## 1788 dislikes 1 4.145765e-05
## 1789 distinguish 1 4.145765e-05
## 1790 divide 1 4.145765e-05
## 1791 diy 1 4.145765e-05
## 1792 doc 1 4.145765e-05
## 1793 documented 1 4.145765e-05
## 1794 dollar 1 4.145765e-05
## 1795 donates 1 4.145765e-05
## 1796 doors 1 4.145765e-05
## 1797 dosen't 1 4.145765e-05
## 1798 dotted 1 4.145765e-05
## 1799 double 1 4.145765e-05
## 1800 downhill 1 4.145765e-05
## 1801 downloading 1 4.145765e-05
## 1802 downright 1 4.145765e-05
## 1803 downside 1 4.145765e-05
## 1804 dpi 1 4.145765e-05
## 1805 dr 1 4.145765e-05
## 1806 drawback 1 4.145765e-05
## 1807 drops 1 4.145765e-05
## 1808 dup 1 4.145765e-05
## 1809 duplicate 1 4.145765e-05
## 1810 duplicates 1 4.145765e-05
## 1811 duplicating 1 4.145765e-05
## 1812 duplicator 1 4.145765e-05
## 1813 dynamically 1 4.145765e-05
## 1814 earlier 1 4.145765e-05
## 1815 early 1 4.145765e-05
## 1816 earmark 1 4.145765e-05
## 1817 east 1 4.145765e-05
## 1818 eblast 1 4.145765e-05
## 1819 eblasts 1 4.145765e-05
## 1820 editor 1 4.145765e-05
## 1821 edits 1 4.145765e-05
## 1822 education 1 4.145765e-05
## 1823 efficiently 1 4.145765e-05
## 1824 eg 1 4.145765e-05
## 1825 eggs 1 4.145765e-05
## 1826 eight 1 4.145765e-05
## 1827 elderly 1 4.145765e-05
## 1828 elegant 1 4.145765e-05
## 1829 elements 1 4.145765e-05
## 1830 eleven 1 4.145765e-05
## 1831 eliminates 1 4.145765e-05
## 1832 elimination 1 4.145765e-05
## 1833 ellington 1 4.145765e-05
## 1834 else's 1 4.145765e-05
## 1835 embed 1 4.145765e-05
## 1836 embedded 1 4.145765e-05
## 1837 emergency 1 4.145765e-05
## 1838 empathetic 1 4.145765e-05
## 1839 employees 1 4.145765e-05
## 1840 empty 1 4.145765e-05
## 1841 en 1 4.145765e-05
## 1842 encounter 1 4.145765e-05
## 1843 encountered 1 4.145765e-05
## 1844 encourage 1 4.145765e-05
## 1845 encrypted 1 4.145765e-05
## 1846 ended 1 4.145765e-05
## 1847 endless 1 4.145765e-05
## 1848 ends 1 4.145765e-05
## 1849 energy 1 4.145765e-05
## 1850 enroll 1 4.145765e-05
## 1851 ensure 1 4.145765e-05
## 1852 entering 1 4.145765e-05
## 1853 entertainment 1 4.145765e-05
## 1854 entire 1 4.145765e-05
## 1855 entries 1 4.145765e-05
## 1856 environmentally 1 4.145765e-05
## 1857 episodic 1 4.145765e-05
## 1858 equally 1 4.145765e-05
## 1859 escalated 1 4.145765e-05
## 1860 esps 1 4.145765e-05
## 1861 establish 1 4.145765e-05
## 1862 everytime 1 4.145765e-05
## 1863 evolve 1 4.145765e-05
## 1864 evolving 1 4.145765e-05
## 1865 exact 1 4.145765e-05
## 1866 examples 1 4.145765e-05
## 1867 exceeds 1 4.145765e-05
## 1868 excited 1 4.145765e-05
## 1869 excludes 1 4.145765e-05
## 1870 execullent 1 4.145765e-05
## 1871 exists 1 4.145765e-05
## 1872 exoenses 1 4.145765e-05
## 1873 exorbitant 1 4.145765e-05
## 1874 expand 1 4.145765e-05
## 1875 expectations 1 4.145765e-05
## 1876 experienced 1 4.145765e-05
## 1877 experiences 1 4.145765e-05
## 1878 experiencing 1 4.145765e-05
## 1879 expiration 1 4.145765e-05
## 1880 explanation 1 4.145765e-05
## 1881 explanatory 1 4.145765e-05
## 1882 explore 1 4.145765e-05
## 1883 explorer 1 4.145765e-05
## 1884 export 1 4.145765e-05
## 1885 exportable 1 4.145765e-05
## 1886 exports 1 4.145765e-05
## 1887 extend 1 4.145765e-05
## 1888 extras 1 4.145765e-05
## 1889 extreme 1 4.145765e-05
## 1890 fab 1 4.145765e-05
## 1891 face 1 4.145765e-05
## 1892 facebook 1 4.145765e-05
## 1893 faced 1 4.145765e-05
## 1894 facility 1 4.145765e-05
## 1895 fade 1 4.145765e-05
## 1896 falling 1 4.145765e-05
## 1897 falls 1 4.145765e-05
## 1898 familiarity 1 4.145765e-05
## 1899 families 1 4.145765e-05
## 1900 fans 1 4.145765e-05
## 1901 fashioned 1 4.145765e-05
## 1902 faults 1 4.145765e-05
## 1903 favorite 1 4.145765e-05
## 1904 feed 1 4.145765e-05
## 1905 feeling 1 4.145765e-05
## 1906 fell 1 4.145765e-05
## 1907 fewer 1 4.145765e-05
## 1908 field 1 4.145765e-05
## 1909 figuring 1 4.145765e-05
## 1910 filled 1 4.145765e-05
## 1911 fills 1 4.145765e-05
## 1912 film 1 4.145765e-05
## 1913 filters 1 4.145765e-05
## 1914 final 1 4.145765e-05
## 1915 financials 1 4.145765e-05
## 1916 fingertips 1 4.145765e-05
## 1917 finicial 1 4.145765e-05
## 1918 finishing 1 4.145765e-05
## 1919 fish 1 4.145765e-05
## 1920 five 1 4.145765e-05
## 1921 flat 1 4.145765e-05
## 1922 flex 1 4.145765e-05
## 1923 floorplans 1 4.145765e-05
## 1924 fluctuates 1 4.145765e-05
## 1925 focusing 1 4.145765e-05
## 1926 folks 1 4.145765e-05
## 1927 follows 1 4.145765e-05
## 1928 fonts 1 4.145765e-05
## 1929 force 1 4.145765e-05
## 1930 forcing 1 4.145765e-05
## 1931 forgotten 1 4.145765e-05
## 1932 formats 1 4.145765e-05
## 1933 forms 1 4.145765e-05
## 1934 forte 1 4.145765e-05
## 1935 frankly 1 4.145765e-05
## 1936 freeze 1 4.145765e-05
## 1937 freezes 1 4.145765e-05
## 1938 freezing 1 4.145765e-05
## 1939 frequent 1 4.145765e-05
## 1940 fresh 1 4.145765e-05
## 1941 friendliness 1 4.145765e-05
## 1942 fro 1 4.145765e-05
## 1943 froze 1 4.145765e-05
## 1944 frustration 1 4.145765e-05
## 1945 fully 1 4.145765e-05
## 1946 fun 1 4.145765e-05
## 1947 functionalities 1 4.145765e-05
## 1948 funds 1 4.145765e-05
## 1949 gain 1 4.145765e-05
## 1950 gaps 1 4.145765e-05
## 1951 gateway 1 4.145765e-05
## 1952 gave 1 4.145765e-05
## 1953 generate 1 4.145765e-05
## 1954 generated 1 4.145765e-05
## 1955 generating 1 4.145765e-05
## 1956 genuine 1 4.145765e-05
## 1957 gibson 1 4.145765e-05
## 1958 gifts 1 4.145765e-05
## 1959 gives 1 4.145765e-05
## 1960 glad 1 4.145765e-05
## 1961 gladstone 1 4.145765e-05
## 1962 glitch 1 4.145765e-05
## 1963 greatly 1 4.145765e-05
## 1964 green 1 4.145765e-05
## 1965 greyed 1 4.145765e-05
## 1966 grrat 1 4.145765e-05
## 1967 guess 1 4.145765e-05
## 1968 guests 1 4.145765e-05
## 1969 guidance 1 4.145765e-05
## 1970 guild 1 4.145765e-05
## 1971 hacked 1 4.145765e-05
## 1972 half 1 4.145765e-05
## 1973 halt 1 4.145765e-05
## 1974 hand 1 4.145765e-05
## 1975 handful 1 4.145765e-05
## 1976 handicap 1 4.145765e-05
## 1977 handles 1 4.145765e-05
## 1978 handling 1 4.145765e-05
## 1979 happening 1 4.145765e-05
## 1980 happily 1 4.145765e-05
## 1981 hasn't 1 4.145765e-05
## 1982 hate 1 4.145765e-05
## 1983 haunting 1 4.145765e-05
## 1984 hears 1 4.145765e-05
## 1985 hearted 1 4.145765e-05
## 1986 heavy 1 4.145765e-05
## 1987 held 1 4.145765e-05
## 1988 helpfulness 1 4.145765e-05
## 1989 hi 1 4.145765e-05
## 1990 hide 1 4.145765e-05
## 1991 him 1 4.145765e-05
## 1992 historical 1 4.145765e-05
## 1993 hit 1 4.145765e-05
## 1994 hitches 1 4.145765e-05
## 1995 holder 1 4.145765e-05
## 1996 holiday 1 4.145765e-05
## 1997 honoring 1 4.145765e-05
## 1998 hoping 1 4.145765e-05
## 1999 horrible 1 4.145765e-05
## 2000 hosted 1 4.145765e-05
## 2001 hosts 1 4.145765e-05
## 2002 hour 1 4.145765e-05
## 2003 html 1 4.145765e-05
## 2004 html5 1 4.145765e-05
## 2005 hunt 1 4.145765e-05
## 2006 hurdles 1 4.145765e-05
## 2007 hurt 1 4.145765e-05
## 2008 i'll 1 4.145765e-05
## 2009 ice 1 4.145765e-05
## 2010 id 1 4.145765e-05
## 2011 id've 1 4.145765e-05
## 2012 idea 1 4.145765e-05
## 2013 ideas 1 4.145765e-05
## 2014 identifiable 1 4.145765e-05
## 2015 identifier 1 4.145765e-05
## 2016 identify 1 4.145765e-05
## 2017 idiosyncrasies 1 4.145765e-05
## 2018 ie 1 4.145765e-05
## 2019 iffy 1 4.145765e-05
## 2020 ignoring 1 4.145765e-05
## 2021 image 1 4.145765e-05
## 2022 imagine 1 4.145765e-05
## 2023 immediacy 1 4.145765e-05
## 2024 immediate 1 4.145765e-05
## 2025 impacts 1 4.145765e-05
## 2026 import 1 4.145765e-05
## 2027 importantly 1 4.145765e-05
## 2028 impressed 1 4.145765e-05
## 2029 impressive 1 4.145765e-05
## 2030 inadequacies 1 4.145765e-05
## 2031 inappropriate 1 4.145765e-05
## 2032 include 1 4.145765e-05
## 2033 included 1 4.145765e-05
## 2034 inclusive 1 4.145765e-05
## 2035 inconsistently 1 4.145765e-05
## 2036 inconvenience 1 4.145765e-05
## 2037 increasingly 1 4.145765e-05
## 2038 incredibly 1 4.145765e-05
## 2039 individuals 1 4.145765e-05
## 2040 industry 1 4.145765e-05
## 2041 inexpensive 1 4.145765e-05
## 2042 inexperience 1 4.145765e-05
## 2043 inherent 1 4.145765e-05
## 2044 inhibits 1 4.145765e-05
## 2045 initiate 1 4.145765e-05
## 2046 injury 1 4.145765e-05
## 2047 inopportune 1 4.145765e-05
## 2048 inquires 1 4.145765e-05
## 2049 insight 1 4.145765e-05
## 2050 insignificant 1 4.145765e-05
## 2051 insisting 1 4.145765e-05
## 2052 inspire 1 4.145765e-05
## 2053 inspired 1 4.145765e-05
## 2054 instances 1 4.145765e-05
## 2055 insult 1 4.145765e-05
## 2056 insurance 1 4.145765e-05
## 2057 insure 1 4.145765e-05
## 2058 intened 1 4.145765e-05
## 2059 intention 1 4.145765e-05
## 2060 interaction 1 4.145765e-05
## 2061 interest 1 4.145765e-05
## 2062 internalized 1 4.145765e-05
## 2063 inticketing 1 4.145765e-05
## 2064 introduced 1 4.145765e-05
## 2065 intuative 1 4.145765e-05
## 2066 intuiative 1 4.145765e-05
## 2067 inundated 1 4.145765e-05
## 2068 invalid 1 4.145765e-05
## 2069 invest 1 4.145765e-05
## 2070 invested 1 4.145765e-05
## 2071 invoice 1 4.145765e-05
## 2072 invoices 1 4.145765e-05
## 2073 invoicing 1 4.145765e-05
## 2074 ios 1 4.145765e-05
## 2075 iphone 1 4.145765e-05
## 2076 ipod 1 4.145765e-05
## 2077 ipods 1 4.145765e-05
## 2078 jobs 1 4.145765e-05
## 2079 joe 1 4.145765e-05
## 2080 joke 1 4.145765e-05
## 2081 jon 1 4.145765e-05
## 2082 jpeg 1 4.145765e-05
## 2083 jr 1 4.145765e-05
## 2084 july 1 4.145765e-05
## 2085 jump 1 4.145765e-05
## 2086 june 1 4.145765e-05
## 2087 kenneth 1 4.145765e-05
## 2088 kinks 1 4.145765e-05
## 2089 kludgy 1 4.145765e-05
## 2090 knowledgable 1 4.145765e-05
## 2091 known 1 4.145765e-05
## 2092 labels 1 4.145765e-05
## 2093 lag 1 4.145765e-05
## 2094 laminated 1 4.145765e-05
## 2095 laptops 1 4.145765e-05
## 2096 larger 1 4.145765e-05
## 2097 largo 1 4.145765e-05
## 2098 launch 1 4.145765e-05
## 2099 layout 1 4.145765e-05
## 2100 layouts 1 4.145765e-05
## 2101 lead 1 4.145765e-05
## 2102 leading 1 4.145765e-05
## 2103 leads 1 4.145765e-05
## 2104 leap 1 4.145765e-05
## 2105 leave 1 4.145765e-05
## 2106 legend 1 4.145765e-05
## 2107 lengthy 1 4.145765e-05
## 2108 lets 1 4.145765e-05
## 2109 levels 1 4.145765e-05
## 2110 liability 1 4.145765e-05
## 2111 lifesaver 1 4.145765e-05
## 2112 lik 1 4.145765e-05
## 2113 liked 1 4.145765e-05
## 2114 likelihood 1 4.145765e-05
## 2115 listens 1 4.145765e-05
## 2116 listing 1 4.145765e-05
## 2117 local 1 4.145765e-05
## 2118 locally 1 4.145765e-05
## 2119 location 1 4.145765e-05
## 2120 logistic 1 4.145765e-05
## 2121 looked 1 4.145765e-05
## 2122 loved 1 4.145765e-05
## 2123 loves 1 4.145765e-05
## 2124 luck 1 4.145765e-05
## 2125 magic 1 4.145765e-05
## 2126 magnetic 1 4.145765e-05
## 2127 maintain 1 4.145765e-05
## 2128 maintenance 1 4.145765e-05
## 2129 maneuver 1 4.145765e-05
## 2130 map 1 4.145765e-05
## 2131 markets 1 4.145765e-05
## 2132 matching 1 4.145765e-05
## 2133 matter 1 4.145765e-05
## 2134 mean 1 4.145765e-05
## 2135 meaning 1 4.145765e-05
## 2136 mechanism 1 4.145765e-05
## 2137 medium 1 4.145765e-05
## 2138 meeting 1 4.145765e-05
## 2139 menial 1 4.145765e-05
## 2140 mentioned 1 4.145765e-05
## 2141 menu 1 4.145765e-05
## 2142 merchandise 1 4.145765e-05
## 2143 merge 1 4.145765e-05
## 2144 messages 1 4.145765e-05
## 2145 michael 1 4.145765e-05
## 2146 migration 1 4.145765e-05
## 2147 mildly 1 4.145765e-05
## 2148 millennial 1 4.145765e-05
## 2149 millie 1 4.145765e-05
## 2150 min 1 4.145765e-05
## 2151 mind 1 4.145765e-05
## 2152 mindless 1 4.145765e-05
## 2153 minor 1 4.145765e-05
## 2154 minute 1 4.145765e-05
## 2155 mirage 1 4.145765e-05
## 2156 misc 1 4.145765e-05
## 2157 miss 1 4.145765e-05
## 2158 missed 1 4.145765e-05
## 2159 mix 1 4.145765e-05
## 2160 mode 1 4.145765e-05
## 2161 modern 1 4.145765e-05
## 2162 modifications 1 4.145765e-05
## 2163 modify 1 4.145765e-05
## 2164 monetarily 1 4.145765e-05
## 2165 money's 1 4.145765e-05
## 2166 monitoring 1 4.145765e-05
## 2167 morning 1 4.145765e-05
## 2168 moved 1 4.145765e-05
## 2169 movement 1 4.145765e-05
## 2170 movie 1 4.145765e-05
## 2171 ms 1 4.145765e-05
## 2172 multitude 1 4.145765e-05
## 2173 music 1 4.145765e-05
## 2174 must 1 4.145765e-05
## 2175 muster 1 4.145765e-05
## 2176 navigating 1 4.145765e-05
## 2177 near 1 4.145765e-05
## 2178 negates 1 4.145765e-05
## 2179 negative 1 4.145765e-05
## 2180 network 1 4.145765e-05
## 2181 neutral 1 4.145765e-05
## 2182 newcomers 1 4.145765e-05
## 2183 newer 1 4.145765e-05
## 2184 next 1 4.145765e-05
## 2185 nights 1 4.145765e-05
## 2186 nine 1 4.145765e-05
## 2187 nineties 1 4.145765e-05
## 2188 nobody 1 4.145765e-05
## 2189 none 1 4.145765e-05
## 2190 nonprofit 1 4.145765e-05
## 2191 notably 1 4.145765e-05
## 2192 notations 1 4.145765e-05
## 2193 note 1 4.145765e-05
## 2194 noted 1 4.145765e-05
## 2195 notices 1 4.145765e-05
## 2196 notifications 1 4.145765e-05
## 2197 notified 1 4.145765e-05
## 2198 notify 1 4.145765e-05
## 2199 nuts 1 4.145765e-05
## 2200 o 1 4.145765e-05
## 2201 obvious 1 4.145765e-05
## 2202 occasion 1 4.145765e-05
## 2203 occasional 1 4.145765e-05
## 2204 occurred 1 4.145765e-05
## 2205 occurring 1 4.145765e-05
## 2206 october 1 4.145765e-05
## 2207 offering 1 4.145765e-05
## 2208 offerings 1 4.145765e-05
## 2209 officer 1 4.145765e-05
## 2210 offline 1 4.145765e-05
## 2211 ongoing 1 4.145765e-05
## 2212 ons 1 4.145765e-05
## 2213 onsale 1 4.145765e-05
## 2214 onus 1 4.145765e-05
## 2215 ook 1 4.145765e-05
## 2216 opens 1 4.145765e-05
## 2217 opera 1 4.145765e-05
## 2218 operate 1 4.145765e-05
## 2219 operational 1 4.145765e-05
## 2220 opportunity 1 4.145765e-05
## 2221 opposite 1 4.145765e-05
## 2222 orchestra 1 4.145765e-05
## 2223 order's 1 4.145765e-05
## 2224 org 1 4.145765e-05
## 2225 org's 1 4.145765e-05
## 2226 oriented 1 4.145765e-05
## 2227 originally 1 4.145765e-05
## 2228 ourselves 1 4.145765e-05
## 2229 outdated 1 4.145765e-05
## 2230 outgoing 1 4.145765e-05
## 2231 outlay 1 4.145765e-05
## 2232 overcharge 1 4.145765e-05
## 2233 overhaul 1 4.145765e-05
## 2234 overly 1 4.145765e-05
## 2235 overpayment 1 4.145765e-05
## 2236 override 1 4.145765e-05
## 2237 oversights 1 4.145765e-05
## 2238 overview 1 4.145765e-05
## 2239 overwhelmed 1 4.145765e-05
## 2240 overwhelming 1 4.145765e-05
## 2241 owners 1 4.145765e-05
## 2242 paid 1 4.145765e-05
## 2243 pain 1 4.145765e-05
## 2244 painless 1 4.145765e-05
## 2245 pains 1 4.145765e-05
## 2246 pair 1 4.145765e-05
## 2247 paired 1 4.145765e-05
## 2248 pandelis 1 4.145765e-05
## 2249 par 1 4.145765e-05
## 2250 paragraph 1 4.145765e-05
## 2251 paralyzing 1 4.145765e-05
## 2252 partnership 1 4.145765e-05
## 2253 parts 1 4.145765e-05
## 2254 pass 1 4.145765e-05
## 2255 passable 1 4.145765e-05
## 2256 paste 1 4.145765e-05
## 2257 patch 1 4.145765e-05
## 2258 patient 1 4.145765e-05
## 2259 pcs 1 4.145765e-05
## 2260 pennies 1 4.145765e-05
## 2261 perceived 1 4.145765e-05
## 2262 perfectly 1 4.145765e-05
## 2263 performing 1 4.145765e-05
## 2264 performs 1 4.145765e-05
## 2265 permissions 1 4.145765e-05
## 2266 personable 1 4.145765e-05
## 2267 personalized 1 4.145765e-05
## 2268 personally 1 4.145765e-05
## 2269 personnel 1 4.145765e-05
## 2270 peterborough 1 4.145765e-05
## 2271 phenomenal 1 4.145765e-05
## 2272 philosophy 1 4.145765e-05
## 2273 phones 1 4.145765e-05
## 2274 photo 1 4.145765e-05
## 2275 physical 1 4.145765e-05
## 2276 pictures 1 4.145765e-05
## 2277 piece 1 4.145765e-05
## 2278 pile 1 4.145765e-05
## 2279 pj 1 4.145765e-05
## 2280 placed 1 4.145765e-05
## 2281 placeholder 1 4.145765e-05
## 2282 places 1 4.145765e-05
## 2283 plain 1 4.145765e-05
## 2284 planned 1 4.145765e-05
## 2285 planning 1 4.145765e-05
## 2286 plans 1 4.145765e-05
## 2287 player 1 4.145765e-05
## 2288 playhouse 1 4.145765e-05
## 2289 plays 1 4.145765e-05
## 2290 pleasant 1 4.145765e-05
## 2291 pleased 1 4.145765e-05
## 2292 pledge 1 4.145765e-05
## 2293 policies 1 4.145765e-05
## 2294 popped 1 4.145765e-05
## 2295 portrays 1 4.145765e-05
## 2296 position 1 4.145765e-05
## 2297 positions 1 4.145765e-05
## 2298 postal 1 4.145765e-05
## 2299 practical 1 4.145765e-05
## 2300 practically 1 4.145765e-05
## 2301 practices 1 4.145765e-05
## 2302 pre 1 4.145765e-05
## 2303 premiere 1 4.145765e-05
## 2304 prepaid 1 4.145765e-05
## 2305 prepared 1 4.145765e-05
## 2306 presented 1 4.145765e-05
## 2307 presenters 1 4.145765e-05
## 2308 president 1 4.145765e-05
## 2309 prevent 1 4.145765e-05
## 2310 preventing 1 4.145765e-05
## 2311 preview 1 4.145765e-05
## 2312 priceless 1 4.145765e-05
## 2313 primary 1 4.145765e-05
## 2314 principle 1 4.145765e-05
## 2315 printer 1 4.145765e-05
## 2316 privacy 1 4.145765e-05
## 2317 processed 1 4.145765e-05
## 2318 produced 1 4.145765e-05
## 2319 profitable 1 4.145765e-05
## 2320 programmer 1 4.145765e-05
## 2321 progress 1 4.145765e-05
## 2322 projects 1 4.145765e-05
## 2323 promise 1 4.145765e-05
## 2324 promoted 1 4.145765e-05
## 2325 promoting 1 4.145765e-05
## 2326 prospect 1 4.145765e-05
## 2327 prosperous 1 4.145765e-05
## 2328 proves 1 4.145765e-05
## 2329 provider 1 4.145765e-05
## 2330 public 1 4.145765e-05
## 2331 publishing 1 4.145765e-05
## 2332 pulls 1 4.145765e-05
## 2333 purcentages 1 4.145765e-05
## 2334 purchaser 1 4.145765e-05
## 2335 purchasers 1 4.145765e-05
## 2336 pushback 1 4.145765e-05
## 2337 putting 1 4.145765e-05
## 2338 q 1 4.145765e-05
## 2339 que 1 4.145765e-05
## 2340 queries 1 4.145765e-05
## 2341 query 1 4.145765e-05
## 2342 quicker 1 4.145765e-05
## 2343 quickness 1 4.145765e-05
## 2344 rain 1 4.145765e-05
## 2345 raised 1 4.145765e-05
## 2346 rancho 1 4.145765e-05
## 2347 random 1 4.145765e-05
## 2348 rank 1 4.145765e-05
## 2349 rapid 1 4.145765e-05
## 2350 rapidly 1 4.145765e-05
## 2351 rare 1 4.145765e-05
## 2352 rated 1 4.145765e-05
## 2353 rates 1 4.145765e-05
## 2354 reaching 1 4.145765e-05
## 2355 reaction 1 4.145765e-05
## 2356 read 1 4.145765e-05
## 2357 readers 1 4.145765e-05
## 2358 readily 1 4.145765e-05
## 2359 reads 1 4.145765e-05
## 2360 reason's 1 4.145765e-05
## 2361 receiving 1 4.145765e-05
## 2362 recharge 1 4.145765e-05
## 2363 recognizes 1 4.145765e-05
## 2364 reconciliation 1 4.145765e-05
## 2365 reconcilliation 1 4.145765e-05
## 2366 recorded 1 4.145765e-05
## 2367 recruit 1 4.145765e-05
## 2368 red 1 4.145765e-05
## 2369 redeeming 1 4.145765e-05
## 2370 redundant 1 4.145765e-05
## 2371 refreshing 1 4.145765e-05
## 2372 refund 1 4.145765e-05
## 2373 registration 1 4.145765e-05
## 2374 regretfully 1 4.145765e-05
## 2375 reinstall 1 4.145765e-05
## 2376 reiterate 1 4.145765e-05
## 2377 relating 1 4.145765e-05
## 2378 relative 1 4.145765e-05
## 2379 release 1 4.145765e-05
## 2380 reliance 1 4.145765e-05
## 2381 reluctant 1 4.145765e-05
## 2382 rely 1 4.145765e-05
## 2383 remained 1 4.145765e-05
## 2384 remaining 1 4.145765e-05
## 2385 remembers 1 4.145765e-05
## 2386 remotely 1 4.145765e-05
## 2387 remove 1 4.145765e-05
## 2388 renegotiating 1 4.145765e-05
## 2389 renewed 1 4.145765e-05
## 2390 renewing 1 4.145765e-05
## 2391 renews 1 4.145765e-05
## 2392 rental 1 4.145765e-05
## 2393 rented 1 4.145765e-05
## 2394 renter 1 4.145765e-05
## 2395 reoccuring 1 4.145765e-05
## 2396 repeatedly 1 4.145765e-05
## 2397 replaying 1 4.145765e-05
## 2398 reply 1 4.145765e-05
## 2399 representatives 1 4.145765e-05
## 2400 represents 1 4.145765e-05
## 2401 reprint 1 4.145765e-05
## 2402 requesting 1 4.145765e-05
## 2403 requirements 1 4.145765e-05
## 2404 reservation 1 4.145765e-05
## 2405 reset 1 4.145765e-05
## 2406 resizing 1 4.145765e-05
## 2407 resolutions 1 4.145765e-05
## 2408 respect 1 4.145765e-05
## 2409 responded 1 4.145765e-05
## 2410 restricted 1 4.145765e-05
## 2411 restrictions 1 4.145765e-05
## 2412 retrieval 1 4.145765e-05
## 2413 retrieve 1 4.145765e-05
## 2414 returning 1 4.145765e-05
## 2415 revamped 1 4.145765e-05
## 2416 rework 1 4.145765e-05
## 2417 rfp 1 4.145765e-05
## 2418 ripped 1 4.145765e-05
## 2419 rock 1 4.145765e-05
## 2420 roll 1 4.145765e-05
## 2421 roof 1 4.145765e-05
## 2422 rooms 1 4.145765e-05
## 2423 routed 1 4.145765e-05
## 2424 royalties 1 4.145765e-05
## 2425 rubs 1 4.145765e-05
## 2426 rudimentary 1 4.145765e-05
## 2427 running 1 4.145765e-05
## 2428 runs 1 4.145765e-05
## 2429 rush 1 4.145765e-05
## 2430 ryerson 1 4.145765e-05
## 2431 sad 1 4.145765e-05
## 2432 safari 1 4.145765e-05
## 2433 salesperson 1 4.145765e-05
## 2434 sanity 1 4.145765e-05
## 2435 satisfactory 1 4.145765e-05
## 2436 satisfies 1 4.145765e-05
## 2437 satisied 1 4.145765e-05
## 2438 saved 1 4.145765e-05
## 2439 saver 1 4.145765e-05
## 2440 saves 1 4.145765e-05
## 2441 savings 1 4.145765e-05
## 2442 savoy 1 4.145765e-05
## 2443 scan 1 4.145765e-05
## 2444 scanning 1 4.145765e-05
## 2445 scavenger 1 4.145765e-05
## 2446 scheduled 1 4.145765e-05
## 2447 schmoe 1 4.145765e-05
## 2448 screens 1 4.145765e-05
## 2449 screwed 1 4.145765e-05
## 2450 script 1 4.145765e-05
## 2451 sea 1 4.145765e-05
## 2452 seact 1 4.145765e-05
## 2453 seact.com 1 4.145765e-05
## 2454 seasoned 1 4.145765e-05
## 2455 secondly 1 4.145765e-05
## 2456 secured 1 4.145765e-05
## 2457 securely 1 4.145765e-05
## 2458 seeing 1 4.145765e-05
## 2459 seemed 1 4.145765e-05
## 2460 selection 1 4.145765e-05
## 2461 selections 1 4.145765e-05
## 2462 seller 1 4.145765e-05
## 2463 sellers 1 4.145765e-05
## 2464 sells 1 4.145765e-05
## 2465 separately 1 4.145765e-05
## 2466 september 1 4.145765e-05
## 2467 server 1 4.145765e-05
## 2468 servers 1 4.145765e-05
## 2469 serviced 1 4.145765e-05
## 2470 servicing 1 4.145765e-05
## 2471 serving 1 4.145765e-05
## 2472 settlement 1 4.145765e-05
## 2473 settlements 1 4.145765e-05
## 2474 setups 1 4.145765e-05
## 2475 severely 1 4.145765e-05
## 2476 share 1 4.145765e-05
## 2477 shift 1 4.145765e-05
## 2478 shit 1 4.145765e-05
## 2479 shooting 1 4.145765e-05
## 2480 shopping 1 4.145765e-05
## 2481 shortcomings 1 4.145765e-05
## 2482 shortfalls 1 4.145765e-05
## 2483 should.the 1 4.145765e-05
## 2484 shouldn't 1 4.145765e-05
## 2485 sight 1 4.145765e-05
## 2486 signficant 1 4.145765e-05
## 2487 simpler 1 4.145765e-05
## 2488 simplistic 1 4.145765e-05
## 2489 simultaneously 1 4.145765e-05
## 2490 singles 1 4.145765e-05
## 2491 sites 1 4.145765e-05
## 2492 sitting 1 4.145765e-05
## 2493 situations 1 4.145765e-05
## 2494 sizes 1 4.145765e-05
## 2495 skill 1 4.145765e-05
## 2496 slcenter 1 4.145765e-05
## 2497 sliding 1 4.145765e-05
## 2498 slight 1 4.145765e-05
## 2499 slowness 1 4.145765e-05
## 2500 snotty 1 4.145765e-05
## 2501 solidly 1 4.145765e-05
## 2502 solved 1 4.145765e-05
## 2503 solves 1 4.145765e-05
## 2504 someone's 1 4.145765e-05
## 2505 somewhat 1 4.145765e-05
## 2506 sooo 1 4.145765e-05
## 2507 sophistication 1 4.145765e-05
## 2508 sorely 1 4.145765e-05
## 2509 sound 1 4.145765e-05
## 2510 spacing 1 4.145765e-05
## 2511 spam 1 4.145765e-05
## 2512 speaking 1 4.145765e-05
## 2513 speaks 1 4.145765e-05
## 2514 specified 1 4.145765e-05
## 2515 speed 1 4.145765e-05
## 2516 spent 1 4.145765e-05
## 2517 split 1 4.145765e-05
## 2518 sponsors 1 4.145765e-05
## 2519 spots 1 4.145765e-05
## 2520 spotty 1 4.145765e-05
## 2521 spouses 1 4.145765e-05
## 2522 stage 1 4.145765e-05
## 2523 stances 1 4.145765e-05
## 2524 standardized 1 4.145765e-05
## 2525 standout 1 4.145765e-05
## 2526 standpoint 1 4.145765e-05
## 2527 stands 1 4.145765e-05
## 2528 state 1 4.145765e-05
## 2529 statistics 1 4.145765e-05
## 2530 steep 1 4.145765e-05
## 2531 stellar 1 4.145765e-05
## 2532 stewardship 1 4.145765e-05
## 2533 stick 1 4.145765e-05
## 2534 stock 1 4.145765e-05
## 2535 stopped 1 4.145765e-05
## 2536 stoppers 1 4.145765e-05
## 2537 stops 1 4.145765e-05
## 2538 storage 1 4.145765e-05
## 2539 straight 1 4.145765e-05
## 2540 strategies 1 4.145765e-05
## 2541 straw 1 4.145765e-05
## 2542 streamline 1 4.145765e-05
## 2543 streamlined 1 4.145765e-05
## 2544 streamlining 1 4.145765e-05
## 2545 strengthen 1 4.145765e-05
## 2546 strengths 1 4.145765e-05
## 2547 stressful 1 4.145765e-05
## 2548 stretch 1 4.145765e-05
## 2549 strictly 1 4.145765e-05
## 2550 struggle 1 4.145765e-05
## 2551 struggling 1 4.145765e-05
## 2552 sub 1 4.145765e-05
## 2553 subscribed 1 4.145765e-05
## 2554 subwscription 1 4.145765e-05
## 2555 successfully 1 4.145765e-05
## 2556 sucks 1 4.145765e-05
## 2557 suffers 1 4.145765e-05
## 2558 sufficient 1 4.145765e-05
## 2559 suggest 1 4.145765e-05
## 2560 suggested 1 4.145765e-05
## 2561 suggestion 1 4.145765e-05
## 2562 suit 1 4.145765e-05
## 2563 suite 1 4.145765e-05
## 2564 suites 1 4.145765e-05
## 2565 suits 1 4.145765e-05
## 2566 summary 1 4.145765e-05
## 2567 supported 1 4.145765e-05
## 2568 supportive 1 4.145765e-05
## 2569 supports 1 4.145765e-05
## 2570 surcharges 1 4.145765e-05
## 2571 surprise 1 4.145765e-05
## 2572 surprised 1 4.145765e-05
## 2573 surprises 1 4.145765e-05
## 2574 survice 1 4.145765e-05
## 2575 survival 1 4.145765e-05
## 2576 suspect 1 4.145765e-05
## 2577 switched 1 4.145765e-05
## 2578 switching 1 4.145765e-05
## 2579 table 1 4.145765e-05
## 2580 tag 1 4.145765e-05
## 2581 tags 1 4.145765e-05
## 2582 taks 1 4.145765e-05
## 2583 tampa 1 4.145765e-05
## 2584 tape 1 4.145765e-05
## 2585 tapping 1 4.145765e-05
## 2586 task 1 4.145765e-05
## 2587 teaches 1 4.145765e-05
## 2588 tells 1 4.145765e-05
## 2589 template 1 4.145765e-05
## 2590 templets 1 4.145765e-05
## 2591 tension 1 4.145765e-05
## 2592 teriiffic 1 4.145765e-05
## 2593 term 1 4.145765e-05
## 2594 terrible 1 4.145765e-05
## 2595 terribly 1 4.145765e-05
## 2596 testing 1 4.145765e-05
## 2597 texas 1 4.145765e-05
## 2598 thankfully 1 4.145765e-05
## 2599 theatre's 1 4.145765e-05
## 2600 theatrical 1 4.145765e-05
## 2601 themselves 1 4.145765e-05
## 2602 therm 1 4.145765e-05
## 2603 thermal 1 4.145765e-05
## 2604 they'll 1 4.145765e-05
## 2605 they've 1 4.145765e-05
## 2606 thorough 1 4.145765e-05
## 2607 thoroughly 1 4.145765e-05
## 2608 threatening 1 4.145765e-05
## 2609 thrilled 1 4.145765e-05
## 2610 throughout 1 4.145765e-05
## 2611 thru 1 4.145765e-05
## 2612 thundertix 1 4.145765e-05
## 2613 ticketed 1 4.145765e-05
## 2614 ticketmaster 1 4.145765e-05
## 2615 tiers 1 4.145765e-05
## 2616 timed 1 4.145765e-05
## 2617 timeline 1 4.145765e-05
## 2618 timely 1 4.145765e-05
## 2619 timeout 1 4.145765e-05
## 2620 tips 1 4.145765e-05
## 2621 token 1 4.145765e-05
## 2622 tomorrow 1 4.145765e-05
## 2623 tonight 1 4.145765e-05
## 2624 tonkenization 1 4.145765e-05
## 2625 tons 1 4.145765e-05
## 2626 tooooo 1 4.145765e-05
## 2627 topic 1 4.145765e-05
## 2628 tory 1 4.145765e-05
## 2629 totally 1 4.145765e-05
## 2630 totals 1 4.145765e-05
## 2631 touch 1 4.145765e-05
## 2632 tours 1 4.145765e-05
## 2633 towards 1 4.145765e-05
## 2634 trained 1 4.145765e-05
## 2635 transferring 1 4.145765e-05
## 2636 treats 1 4.145765e-05
## 2637 tremendously 1 4.145765e-05
## 2638 tricia 1 4.145765e-05
## 2639 troubles 1 4.145765e-05
## 2640 troubleshooting 1 4.145765e-05
## 2641 trump 1 4.145765e-05
## 2642 trust 1 4.145765e-05
## 2643 tues 1 4.145765e-05
## 2644 turn 1 4.145765e-05
## 2645 tutorial 1 4.145765e-05
## 2646 typically 1 4.145765e-05
## 2647 ugly 1 4.145765e-05
## 2648 umbrella 1 4.145765e-05
## 2649 un 1 4.145765e-05
## 2650 unavailable 1 4.145765e-05
## 2651 unaware 1 4.145765e-05
## 2652 unbelievable 1 4.145765e-05
## 2653 unconscionable 1 4.145765e-05
## 2654 unexpected 1 4.145765e-05
## 2655 unfilled 1 4.145765e-05
## 2656 unfixed 1 4.145765e-05
## 2657 unhelpful 1 4.145765e-05
## 2658 unique 1 4.145765e-05
## 2659 unlikely 1 4.145765e-05
## 2660 unlock 1 4.145765e-05
## 2661 unneeded 1 4.145765e-05
## 2662 unpractical 1 4.145765e-05
## 2663 unreliable 1 4.145765e-05
## 2664 unresponsive 1 4.145765e-05
## 2665 unsatisfactory 1 4.145765e-05
## 2666 unstable 1 4.145765e-05
## 2667 unsubscribed 1 4.145765e-05
## 2668 unusable 1 4.145765e-05
## 2669 unwieldy 1 4.145765e-05
## 2670 unwillingness 1 4.145765e-05
## 2671 upcoming 1 4.145765e-05
## 2672 updated 1 4.145765e-05
## 2673 uploaded 1 4.145765e-05
## 2674 upon 1 4.145765e-05
## 2675 urgency 1 4.145765e-05
## 2676 urgent 1 4.145765e-05
## 2677 usage 1 4.145765e-05
## 2678 usefulness 1 4.145765e-05
## 2679 uswer 1 4.145765e-05
## 2680 utilizing 1 4.145765e-05
## 2681 valid 1 4.145765e-05
## 2682 valued 1 4.145765e-05
## 2683 valunable 1 4.145765e-05
## 2684 vein 1 4.145765e-05
## 2685 vendiini's 1 4.145765e-05
## 2686 versatile 1 4.145765e-05
## 2687 vice 1 4.145765e-05
## 2688 video's 1 4.145765e-05
## 2689 viewpoint 1 4.145765e-05
## 2690 virtually 1 4.145765e-05
## 2691 visual 1 4.145765e-05
## 2692 visually 1 4.145765e-05
## 2693 volunteer 1 4.145765e-05
## 2694 w 1 4.145765e-05
## 2695 walletini 1 4.145765e-05
## 2696 waning 1 4.145765e-05
## 2697 wanted 1 4.145765e-05
## 2698 warn 1 4.145765e-05
## 2699 washington 1 4.145765e-05
## 2700 wasted 1 4.145765e-05
## 2701 watching 1 4.145765e-05
## 2702 we'll 1 4.145765e-05
## 2703 weakest 1 4.145765e-05
## 2704 webmaster 1 4.145765e-05
## 2705 webpages 1 4.145765e-05
## 2706 west 1 4.145765e-05
## 2707 whatsoever 1 4.145765e-05
## 2708 whistles 1 4.145765e-05
## 2709 who's 1 4.145765e-05
## 2710 wife 1 4.145765e-05
## 2711 wilsonville 1 4.145765e-05
## 2712 wireless 1 4.145765e-05
## 2713 wise 1 4.145765e-05
## 2714 wishing 1 4.145765e-05
## 2715 wonderfully 1 4.145765e-05
## 2716 wonders 1 4.145765e-05
## 2717 wont 1 4.145765e-05
## 2718 words 1 4.145765e-05
## 2719 workaround 1 4.145765e-05
## 2720 workflow 1 4.145765e-05
## 2721 world 1 4.145765e-05
## 2722 worst 1 4.145765e-05
## 2723 worthwhile 1 4.145765e-05
## 2724 written 1 4.145765e-05
## 2725 x 1 4.145765e-05
## 2726 y 1 4.145765e-05
## 2727 yeah 1 4.145765e-05
## 2728 year's 1 4.145765e-05
## 2729 yearly 1 4.145765e-05
## 2730 yield 1 4.145765e-05
## 2731 yo 1 4.145765e-05
## 2732 you've 1 4.145765e-05
## 2733 yourself 1 4.145765e-05
## 2734 youth 1 4.145765e-05
## 2735 zipcode 1 4.145765e-05
Next we will calculate the skipgram probabilities, how often we find a word near another word. To do this we will create a sliding window of words around a word and then use pairwise_count to count cooccuring pairs within each sliding window. Essentially we will be doing this P(word1, word2) /P(word1)/ P(word2). We’ll take a window of 8 words for each sliding window. I reached this number after some experimentation.
skipgrams <- comments %>%
unnest_tokens(ngram, Comment, token = "ngrams", n = 8) %>%
mutate(ngramID = row_number()) %>%
unite(skipgramID, docID, ngramID) %>%
unnest_tokens(word, ngram)
Next we do a pairwise count of the terms and find the relative probabilities.
skipgram_probs <- skipgrams %>%
pairwise_count(word, skipgramID, sort = TRUE, diag = TRUE) %>%
mutate(p = n /sum(n))
Normalized skipgram probability
normalized_prob <- skipgram_probs %>%
filter(n > 20) %>%
rename(word1 = item1, word2 = item2) %>%
left_join(unigram_probs %>%
select(word1 = word, p1 = p),
by = "word1") %>%
left_join(unigram_probs %>%
select(word2 = word, p2 = p),
by = "word2") %>%
mutate(p_together = p / p1 / p2)
Just with this information we can try and find some word associations - which words are most closely associated with service
normalized_prob %>%
filter(word1 == "service") %>%
arrange(-p_together)
## # A tibble: 39 × 7
## word1 word2 n p p1 p2 p_together
## <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
## 1 service service 718 0.000675 0.0116 0.0116 5.01
## 2 service customer 336 0.000316 0.0116 0.00949 2.87
## 3 service excellent 37 0.0000348 0.0116 0.00153 1.95
## 4 service their 25 0.0000235 0.0116 0.00149 1.36
## 5 service product 40 0.0000376 0.0116 0.00269 1.20
## 6 service great 116 0.000109 0.0116 0.00833 1.13
## 7 service good 48 0.0000451 0.0116 0.00369 1.05
## 8 service your 49 0.0000460 0.0116 0.00398 0.997
## 9 service but 66 0.0000620 0.0116 0.00593 0.901
## 10 service get 25 0.0000235 0.0116 0.00257 0.787
## # ℹ 29 more rows
How about customer
normalized_prob %>%
filter(word1 == "customer") %>%
arrange(-p_together)
## # A tibble: 31 × 7
## word1 word2 n p p1 p2 p_together
## <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
## 1 customer customer 588 0.000553 0.00949 0.00949 6.13
## 2 customer service 336 0.000316 0.00949 0.0116 2.87
## 3 customer by 22 0.0000207 0.00949 0.00108 2.02
## 4 customer excellent 25 0.0000235 0.00949 0.00153 1.61
## 5 customer support 109 0.000102 0.00949 0.00775 1.39
## 6 customer great 78 0.0000733 0.00949 0.00833 0.927
## 7 customer good 31 0.0000291 0.00949 0.00369 0.832
## 8 customer very 43 0.0000404 0.00949 0.00556 0.766
## 9 customer friendly 24 0.0000226 0.00949 0.00369 0.644
## 10 customer and 208 0.000195 0.00949 0.0349 0.589
## # ℹ 21 more rows
How about the negative qualifier not
normalized_prob %>%
filter(word1 == "not") %>%
arrange(-p_together)
## # A tibble: 79 × 7
## word1 word2 n p p1 p2 p_together
## <chr> <chr> <dbl> <dbl> <dbl> <dbl> <dbl>
## 1 not not 1314 0.00123 0.00866 0.00866 16.4
## 2 not did 50 0.0000470 0.00866 0.000497 10.9
## 3 not sure 26 0.0000244 0.00866 0.000332 8.50
## 4 not enough 23 0.0000216 0.00866 0.000332 7.52
## 5 not does 56 0.0000526 0.00866 0.000829 7.33
## 6 not fixed 26 0.0000244 0.00866 0.000415 6.80
## 7 not flexible 27 0.0000254 0.00866 0.000497 5.89
## 8 not i'm 32 0.0000301 0.00866 0.000663 5.23
## 9 not may 23 0.0000216 0.00866 0.000497 5.01
## 10 not perfect 41 0.0000385 0.00866 0.000912 4.88
## # ℹ 69 more rows
Next we want to perform matrix factorization, cast to a matrix. This produces a matrix of m x m size with most of values being 0.
pmi_matrix <- normalized_prob %>%
mutate(pmi = log10(p_together)) %>%
cast_sparse(word1, word2, pmi)
Next, produce a sparse matrix to reduce dimesionality.
pmi_svd <- irlba(pmi_matrix, 256, maxit = 1e3)
Next we get the word vectors
word_vectors <- pmi_svd$u
rownames(word_vectors) <- rownames(pmi_matrix)
Here is function to tidy the output
search_synonyms <- function(word_vectors, selected_vector) {
similarities <- word_vectors * selected_vector %>%
tidy() %>%
as_tibble() %>%
rename(token = .rownames,
similarity = unrowname.x.)
similarities %>%
arrange(-similarity)
}
Graph Analysis
Next, we will look at the bigrams again, but this time create a graph of co-occuence and other similar graph operations. Start by creating bigrams and creating a graph of linked words.
bigram_graph <- comments %>%
unnest_tokens(bigram ,Comment, token = "ngrams", n = 2) %>%
separate(bigram, c("word1", "word2"), sep = " " ) %>%
anti_join(stop_words, by = c("word1" = "word")) %>%
anti_join(stop_words, by = c("word2" = "word")) %>%
group_by(cat) %>%
count(word1, word2, sort = TRUE) %>%
select(word1, word2, cat, n) %>%
filter(n>2) %>%
as_tbl_graph()
## Warning in graph_from_data_frame(x, directed = directed): In `d' `NA' elements
## were replaced with string "NA"
Now let’s plot out the central themes here.
Centrality of words
bigram_graph %>%
mutate(centrality = centrality_degree()) %>%
ggraph(layout = 'kk') +
geom_edge_link() +
geom_node_point(aes(size = centrality, color = centrality)) +
geom_node_text(aes(label = name), repel = TRUE) +
theme_graph() +
theme(legend.position = "none")
## Warning: Using the `size` aesthetic in this geom was deprecated in ggplot2 3.4.0.
## ℹ Please use `linewidth` in the `default_aes` field and elsewhere instead.
## This warning is displayed once every 8 hours.
## Call `lifecycle::last_lifecycle_warnings()` to see where this warning was
## generated.
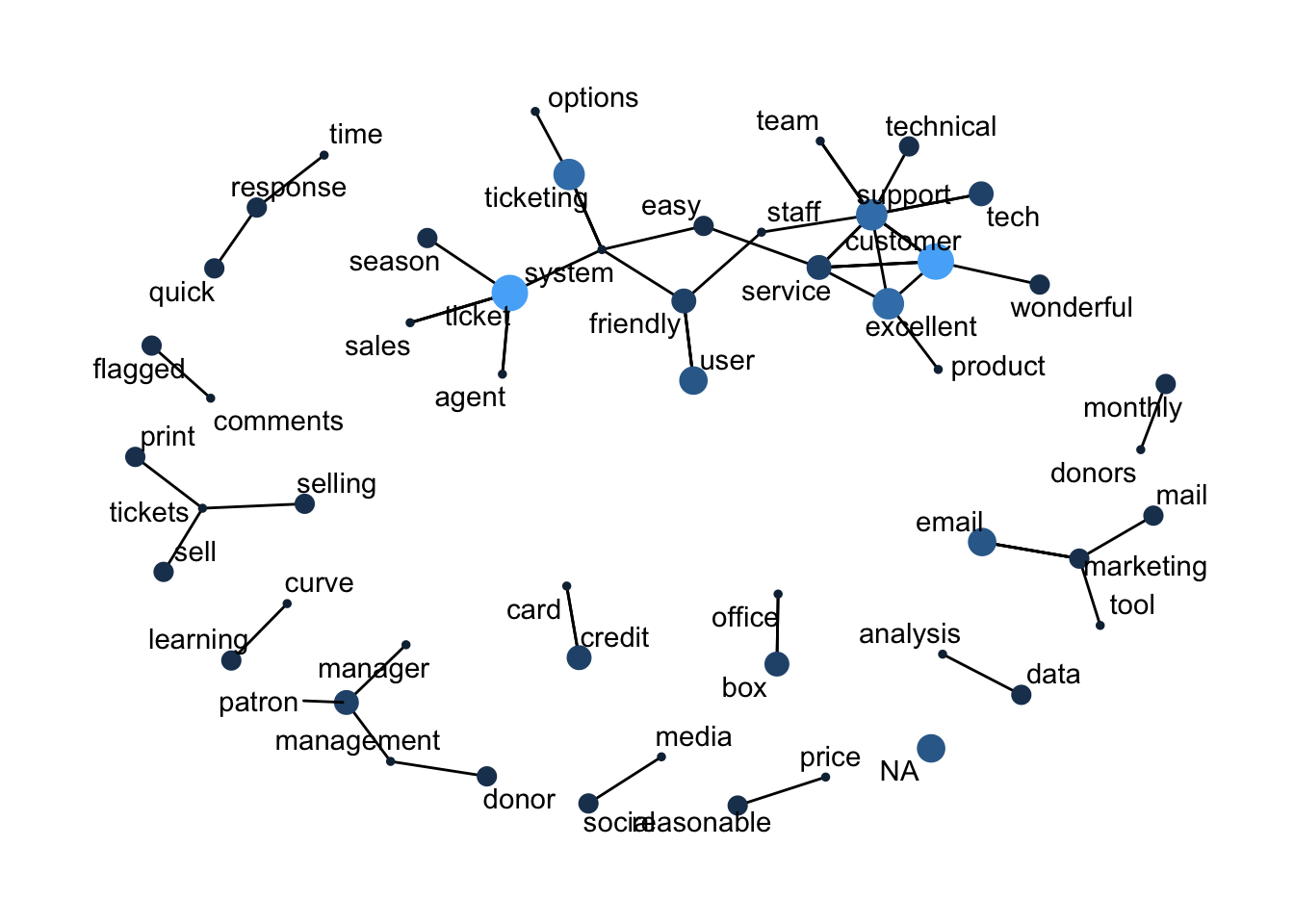
Clusters
bigram_graph %>%
mutate(community = as.factor(group_infomap())) %>%
ggraph(layout = 'kk') +
geom_edge_link(aes(alpha = ..index..), show.legend = FALSE) +
geom_node_point(aes(colour = community), size = 5) +
geom_node_text(aes(label = name), repel = TRUE) +
theme_graph()
## Warning: The dot-dot notation (`..index..`) was deprecated in ggplot2 3.4.0.
## ℹ Please use `after_stat(index)` instead.
## This warning is displayed once every 8 hours.
## Call `lifecycle::last_lifecycle_warnings()` to see where this warning was
## generated.
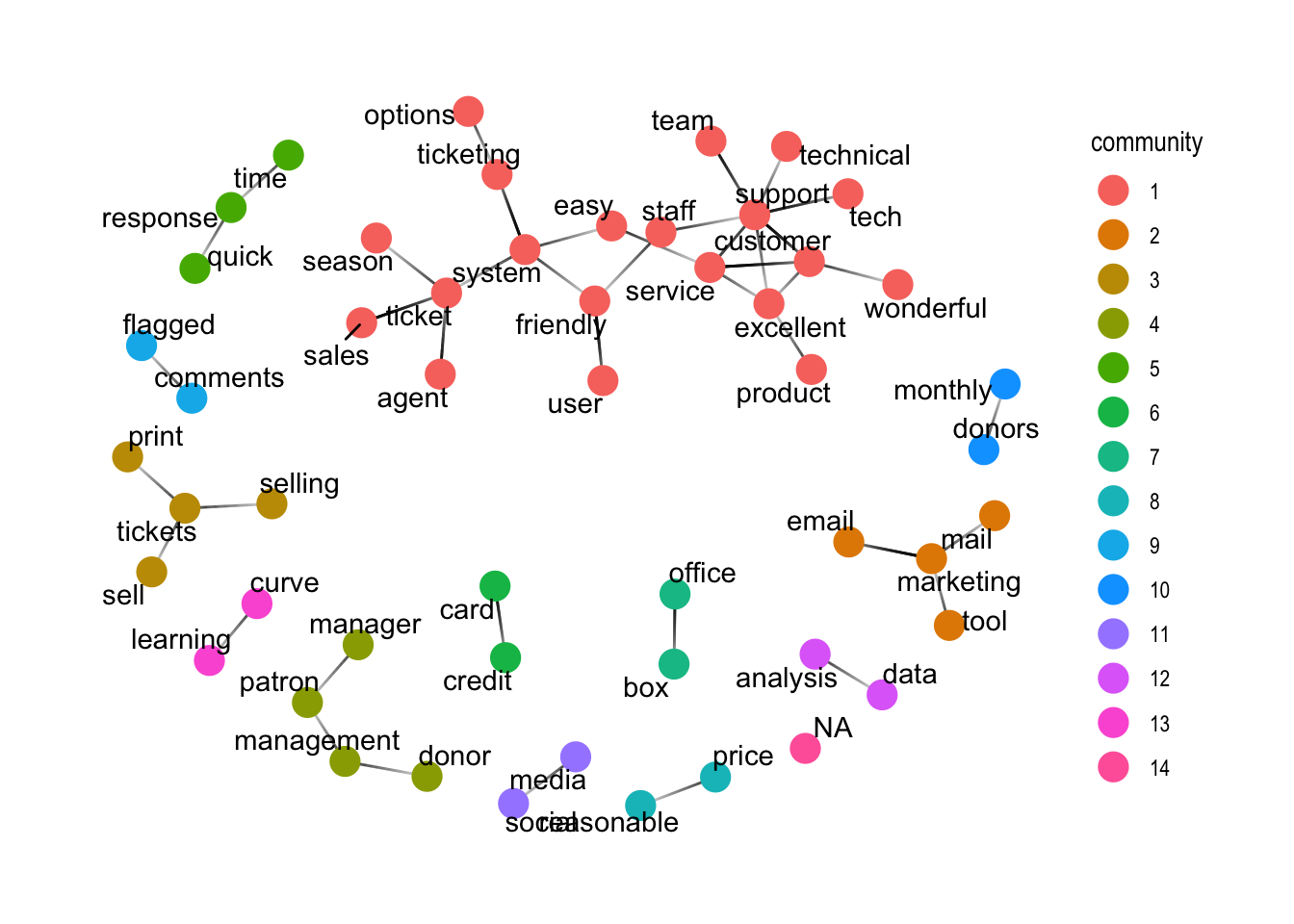
Final Thoughts
In these two posts we explored NPS quite deeply. We saw that the score had moved up consistently over the years, we saw that respondents clearly gave higher scores on certain days of the week, the platform is serving certain types of business better than others, comments are generally positive and customer service is loved accross the board but is also blamed for system shortcomings. We had a limted corpus of comments and having more would have helped with LDA and topic modeling.